Concatenation and Combining Strings in PHP
How to combine two or more strings into one big string in PHP.
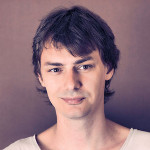
By. Jacob
Edited: 2021-02-10 15:44
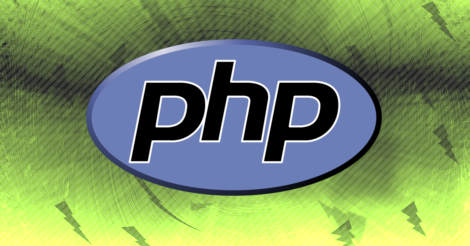
A common desire of a PHP developer is to combine several strings so they become one string. In programming, this is also known as string concatenation.
To concatenate a string in PHP, we will usually be using (.) periods. While generally easy, it gets harder when you want to use a mix of functions and strings. Using a function in a concatenated string allows us to use the function-output in-place, without first having to assign it to a variable.
Note. It is a good practice to avoid using extra variables that are not needed by the application.
The below is a very simple string, assembled using dots (periods):
$name = 'Quackmore Duck';
echo 'My name is ' . $name . ' and I am the father to Donald Duck.';
The same approach works when concatenating variables:
$name = 'Quackmore Duck';
$output = 'My name is ' . $name . ' and I am the father to Donald Duck.';
echo $output;
This is the preferred approach to concatenation.
String concatenation with echo
When echo'ing content, we could also use a comma as separator. Personally, I hate this, because it just adds another way of doing the same thing, and the same syntax can not be used for variables.
echo 'This ', 'is a', ' concatenated string';
However, if we tried concatenating a variable:
$name = 'Rasmus Lerdorf';
$output = 'My name is ', $name, ' and I am the inventor of PHP.';
echo $output;
This would just result in a parse error:
PHP Parse error: syntax error, unexpected ',' in /var/www/string_concatenation.php on line 3
Concatenation with functions
Using functions mixed with other functions or strings is also possible using the same syntax:
echo 'Today\'s date is: ' . date('Y-m-d H:i:s') . ' and the sun is shining.';
You can also concatenate multiple functions next to each other:
echo 'This is the date: ' . date('Y') . date('m') . date('d');
Tell us what you think: