PHP: How to Remove Duplicated Values in Arrays
To get rid of duplicated entries in arrays we may either create our own function, or we can use the build-in array_unique(); function, both of which is demonstrated in this tutorial.
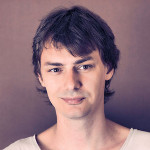
By. Jacob
Edited: 2020-09-30 09:15
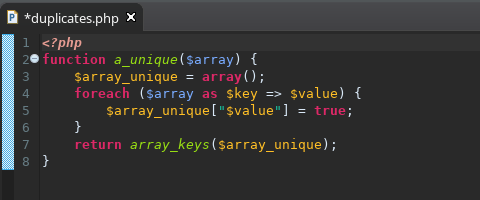
To remove duplicated values from an array in PHP, we may use a combination of isset and array keys. This method of finding duplicates is generally very fast.
This works because any binary string will work as an array key. Armed with this knowledge, we can easily determine if something already exists in an array with a simple algorithm like this one:
function a_unique($array) {
$array_unique = array();
foreach ($array as $key => $value) {
$array_unique["$value"] = true;
}
return array_keys($array_unique);
}
$array_made_unique = a_unique($array);
print_r($array_made_unique);
Removing duplicates with PHP
When looking at a problem where you have duplicated entries in an array, it is usually because a function gave you duplicated results, possible from a poorly designed database query; ideally, you should try to fix your query rather than work around the problem from PHP — if it is a query problem, then solving it from PHP will just unnecessarily complicate your code.
There are circumstances where you will need to remove duplicates in PHP; Personally, I have found that array keys can sometimes be used very effectively to solve the problem in cases where you are using a loop to work with the array data.
I have really come to like the simplicity of the foreach loop. Let us try and use a foreach to find duplicates:
function a_unique($array) {
$array_unique = array();
foreach ($array as $key => $value) {
$array_unique["$value"] = true;
}
return array_keys($array_unique);
}
Now we just have to call the a_unique(); function when we want to remove duplicated values from an array:
$array_duplicates_removed = a_unique($some_array);
foreach ($array_duplicates_removed as &$value) {
echo $value . '<br>';
}
PHP also has its own build-in function to remove duplicates, the array_unique() function.
$input = array("a" => "green", "red", "b" => "green", "blue", "red");
$result = array_unique($input);
print_r($result);
Links
- PHP: array_unique – php.net
Tell us what you think: