PHP: Header
The PHP header function is used to send custom headers and response codes in response to HTTP requests.
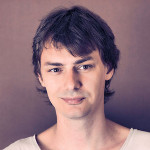
By. Jacob
Edited: 2020-12-01 14:51
The PHP Header function is used to send HTTP Headers in response to a HTTP request, typically coming from a browser. Headers must be sent before any output is sent to the browser.
To send a simple header response, such as that used for permanent redirects, we may use the below:
header('HTTP/1.1 301 Moved Permanently');
header('Location: http://beamtic.com/new-url');
From PHP 5.4 and up, you should use http_response_code() to set response codes. This is much easier, since you do not have to determine the protocol used by the client, and it eliminates the risk of typos in your code:
http_response_code(301);
header('Location: http://beamtic.com/new-url');
Response Codes
Status codes are used to tell the browser if the requested resource exists, has been modified, or if it has been moved to another location.
The standard response for a PHP script is 200 OK, but we can change this using the PHP header function. We would typically do this for caching purposes, or to redirect the request in case the resource was moved. To send a 200 ok message we can do like this:
header('HTTP/1.1 200 OK');
It is also a useful way to deliver error response codes which are easily understood by other services. This could be 404 Not Found or the infamous 500 Internal Server Error. Here are some examples:
- header('HTTP/1.1 404 Not Found')
- header('HTTP/1.1 403 Forbidden')
- header('HTTP/1.1 400 Bad Request')
- header('HTTP/1.1 500 Internal Server Error')
- header('HTTP/1.1 301 Moved Permanently')
Header Redirect
Redirects are performed with the HTTP Location Header, usually in combination with the relevant status code, such as the 303 See Other status code.
header(set_protocol() . ' 303 See Other');
header('Location: http://www.example.com/');
The function uses the SERVER_PROTOCOL variable to determine the protocol used by the client:
function set_protocol() {
$supported_protocols = array(
'HTTP/2.0' => true,
'HTTP/1.1' => true,
'HTTP/1.0' => true,
);
$protocol = $_SERVER["SERVER_PROTOCOL"];
if (!isset($supported_protocols["$protocol"])) {
$protocol = 'HTTP/1.0';
}
return $protocol;
}
This function will use HTTP/1.0 as a fall-back for the response, if the protocol is not found in the $supported_protocols array; this way we may also reject malformed requests or unrecognized protocols.
Note that the HTTP/1.0 spec does not require a host header to be sent by the client — instead you might want to show an error message to the user when the protocol is not recognized.
You can remove the protocols not used by your application by removing it from the array. Also, you might want to consider using http_response_code instead, since the protocol will then be set automatically by PHP.
See also
- HTTP Response Codes – a list of response codes.
Links
- header - php.net
Tell us what you think: