Checking if a variable is a string in PHP
How to check if a variable is of the string type in PHP.
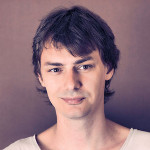
By. Jacob
Edited: 2021-02-14 01:50
To check if a variable is a string, we can use the is_string function; this function will return true if the input is a string, and false otherwise.
To use the is_string function we may write a if statement:
$my_name = 'Thomas';
if (is_string($my_name)) {
echo '$my_name is a string.';
}
When using is_string(); in a function to validate a parameter, we can do like this:
function ($input_string) {
if (!is_string($input_string)) {
echo 'Invalid input. Only string types is allowed!';
exit();
}
// Return the string if valid
return 'Success: <pre>'.$input_string.'</pre> was valid.';
}
This is also a useful way to validate the type of a input variable or function argument; but type hinting is a better option.
Type hinting vs type checking
Rather than manually validating data types, we can also rely on type hinting in PHP 7. To do so we simply "hint" the type of the function or method argument:
function (string $input_string) {
return 'Success: <pre>'.$input_string.'</pre> was valid.';
}
If the input was not valid, an E_ERROR "Uncaught TypeError" will be thrown, without you having to do any validation yourself.
If you feed the function a integer instead of string, PHP will not output any error. This is due to PHPs automatic type juggling, which considers '500' to be the same as 500. In most cases, this should not cause any problems, but if you want to enable strict type checking, you can fix it by including the following at the top of your index.php:
<?php declare(strict_types=1);
Tell us what you think: