Checking if a variable is a number in PHP
Tutorial showing how to check if a variable is a number in PHP
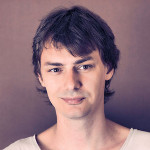
By. Jacob
Edited: 2021-02-14 01:51
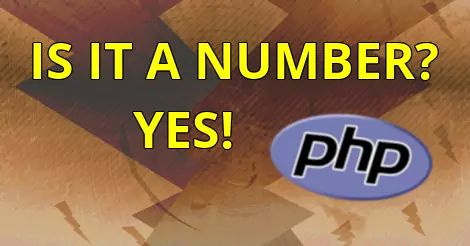
To check if a variable is a number, we could use the is_int function, but we can also use a regular expression with preg_match if we wanted to do more complex validation.
The is_int() function works because an int, or integer, is a number.
Checking it is a simple matter of using a if statement:
$number = 2323;
if(is_int($number)) {
echo 'Dealing with a number.';
} else {
echo 'Not a number!';
}
Another way to check and validate numbers, if you want the be more sophisticated, is to use a regular expression. The following example uses preg_match to perform, basically, the same validation without checking the type of the variable:
$number = 33;
if(preg_match('|^[0-9]+$|', $number)) {
echo 'Dealing with a number.';
} else {
echo 'Not a number!';
}
This regular expression, ^[0-9]+$, basically checks for a number with one or more digits (+), and allows for everything between 0 and 9 to be used in the variable. Finally, the funny hat ^ symbol marks the beginning of the variable, while the dollar sign $ marks the end.
If you leave out the start or the end mark in a regular expression, you could end up allowing (matching) more input than you expect.
At first it might not look like there is a difference between using is_int and preg_match, but there actually is! If you use preg_match(), you will not be validating the type of the variable. Instead, both a number declared as a string, $number = '44'; , and a number declared as an integer, $number = 44;, will be accepted. If instead you use is_int(), only the type of the variable will be checked.
As with many things in PHP, there is many ways to to accomplish the same end goals, and this is also the case for checking if a variable is a number. Some ways are less known than other ways. You can also check a variable using Type casting, it works by wrapping int in parentheses in front of the variable, and then using the type comparison operator (===): The above should result in: Not a number!, since the original variable was declared as a string. Not that this is recommended, but it is useful to know about type casting.
The difference
Using Type Casting
$number = '33';
if ($number === (int) $number) {
echo 'Dealing with a number.';
} else {
echo 'Not a number!';
}
Tell us what you think: