Counting to 100.000 in PHP and C++
How much faster is C++ than PHP to increment and display a counter in a loop?
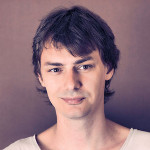
By. Jacob
Edited: 2021-06-29 13:13
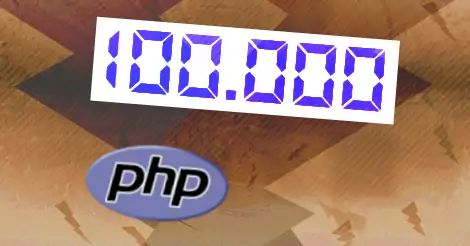
I was wondering how fast I would be able to count to 100.000 using a PHP script while echo'ing each count to the terminal.
I was actually assuming it would take less than a second, but my expectation was clearly way off. I even tried using different loop types, such as while, for and do ... while to see if one would be faster than others — this did not really improve things much.
Note. Changing the loop-type is considered a micro-optimization, and it rarely yields any meaningful performance gains.
So, I finally settled with using a for loop like this:
for ($i=0; $i < 100000; ++$i) {
echo $i . "\n";
}
On my old i3 laptop this executed in about 3 seconds. This was a surprise — I think this is extremely slow for addition and echo inside a loop.
If we compare this to a similar loop written in c++ and compiled to an executable, then it executes just about 7 times faster, at 0.4 seconds:
#include <iostream>
using namespace std;
int main()
{
int count;
for (int count = 0; count < 100000; count++)
{
cout << count << "\n";
}
return 0;
}
How was the test performed
The script was executed from a terminal using the following command:
time php this.php
I even tried it with opcache and JIT enabled:
time php -dopcache.enable_cli=1 -dopcache.jit_buffer_size=100M this.php
Enabling opcache/JIT does not appear to effect the execution time much in this case.
Testing the c++ code
The c++ code was compiled using this command:
c++ -o test test.cpp
And executed with the time command:
time ./test
Conclusion
The test results in this article can give you a rough idea about how much faster compiled C++ is compared to PHP code — but keep in mind that this is only a very basic example, and can it can not be transferred directly to more complex operations.
There may be invisible things happening under the hood that makes this operation slower from PHP than from a compiled C++ program. One thing that might influence the result a little is that it also takes a tiny amount of time to invoke PHP to execute the script vs just running a program directly, but my guess is that this is miniscule considering the fact that it only happens once, before executing the script.
Finally, I also suspect the result might vary depending on CPU used, local settings, and even whether the script is executed through a web server or as a CLI-script.
Tell us what you think: