PHP: Using Echo, Print, Print_r and Var_dump
This tutorial explains how to use the print_r and var_dump functions, and the difference between echo and print.
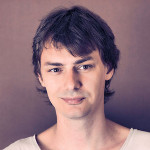
By. Jacob
Edited: 2022-03-25 08:37
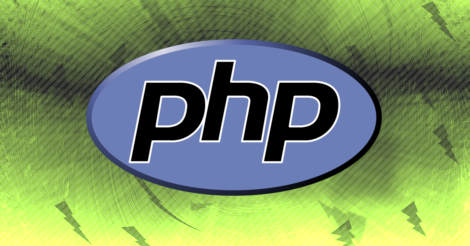
In PHP, we have build-in methods to output content, such as echo and print these methods each have their own use cases.
echo is a language-construct in PHP that allows us to output data in-place. It should not be used before sending headers to the browser, as this would result in a headers already sent... error. The below example shows how to concatenate a few strings and the output of a function using echo:
echo 'Today\'s date is: ' . date('Y-m-d H:i:s') . ' and it is a wonderful day!' . PHP_EOL;
Another way to concatenate strings using commas instead of periods with echo, and which does not work with print, would be like this:
echo 'Today\'s date: ', date('Y-m-d H:i:s'), PHP_EOL;
print is also a language construct (not a function), and is slightly slower than echo, so usually people will prefer to use echo. The speed difference is insignificant, and there might still be times where you want to use print instead. Print takes a single parameter, and will always return "1".
Print_r and Var_dump
In addition to the before mentioned language constructs, we also got functions such as print_r and var_dump. Personally I only use these when I am debugging something, as they can be very useful when trying to understand the nature of the data you are working with.
When used, these functions are often followed by exit in order to output the content of a variable directly to the client, and not do anything else.
Print_r is useful to output the contents of arrays in a fairly readable plain text format:
$the_duck_family = array('Scrooge McDuck', 'Donald Duck', 'Huey', 'Dewey', 'Louie');
print_r($the_duck_family);
exit(); // Exit before other code can execute
Output:
Array
(
[0] => Scrooge McDuck
[1] => Donald Duck
[2] => Huey
[3] => Dewey
[4] => Louie
)
Using var_dump we will also get information about the data types and lengths of the variable:
var_dump($the_duck_family);
exit(); // Exit before other code can execute
Output:
array(5) {
[0]=>
string(14) "Scrooge McDuck"
[1]=>
string(11) "Donald Duck"
[2]=>
string(4) "Huey"
[3]=>
string(5) "Dewey"
[4]=>
string(5) "Louie"
}
Output objects with print_r and var_dump
Both print_r and var_dump will work on objects as well, var_dump being the more detailed pick:
class the_hallo_class
{
private $name = 'Donald Duck';
public function say_hallo()
{
return 'Hallo ' . $this->name;
}
}
$the_hallo_class = new the_hallo_class();
var_dump($the_hallo_class);exit();
Output:
object(the_hallo_class)#1 (1) {
["name":"the_hallo_class":private]=>
string(11) "Donald Duck"
}
If we instead use print_r, the output will be:
the_hallo_class Object
(
[name:the_hallo_class:private] => Donald Duck
)
Tell us what you think: