How to Get The Value of an Input Field in JavaScript
This article explains how to get the value of an input field in JavaScript, and gives basic advice on accessibility of using JavaScript to make custom buttons and forms.
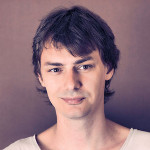
By. Jacob
Edited: 2019-11-03 04:44
JavaScript is not my favorite language, and I rarely use it unless I really have to – or unless it makes sense in the context. Some people like to use it for everything, even generating the main content/HTML on their pages!
Recently I had to return the content of the value attribute on an HTML input element using JavaScript. Not surprisingly, .innerHTML will not work for this purpose. You have to use .value instead.
I was first trying to use .innerHTML, and quickly realized, based on the method name alone, that this only works when working with the content of elements, and not attribute values. Using .value, however, is just as easy:
let input_value = document.getElementById("some_unique_id").value;
document.getElementById("some_unique_id").value = 'Change the value content to this :-D';
The above example shows both how to fetch the value, and to change it. As you can see, there is not much difference in how you use .innerHTML and .value when coding.
A warning about using JavaScript
Using JavaScript can sometimes make your website less accessible. So, it is important you understand the impact it might have on User Experience (UX), as well as the problems you might run into with search engines.
Search engines will typically not need to index HTML forms and input elements, but they do need to index the main content on pages. The main content, if text, should be placed using semantic sectioning elements and p elements from the server-side. This requirement may change as search engines get better at indexing JS. But, as of the writing of this article, Google is still pretty bad at handling JavaScript while other search engines might not crawl it at all.
Another problem that few seem to consider is that you can not easily tell search engines when your content was last updated. By using a server-side language, however, this can be done in the HTTP headers.
The worst problem, depending on how you look at things, is the negative impact JavaScript/AJAX may have on User Experience (depending on how you use it). If you use elements for something they are not intended, this might give problems with UX. A very common example, which can cause problems, would be using a div instead of a button element.
JavaScript and semantic HTML elements
Elements have default behaviors that are not easily changed, unless you know how to do it. Even then, why would you? When you have perfectly good, semantic elements, already serving the function you want?
If you must use custom elements, consider changing the role and tabindex attributes on the element to make it more accessible.
<div role="button" tabindex="0">Post Comment</div>
role="button" is used to turn your element into a button, which should expose it as a button in user agents. Screenreaders should announce it as a button to the user, and it should work exactly like a button.
This is assuming you have the correct JavaScript event handlers in place. Both clicking and pressing enter should activate the button to make it accessible, and you should also be able to navigate using tab.
tabindex makes the element focusable, and part of the taborder in the document. This is used to control the order that elements gain focus when using the tabulator key on a keyboard to navigate.
Tell us what you think: