Making a Fade Effect in JavaScript
How to make a simple fade effect without the use of libraries.
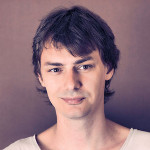
By. Jacob
Edited: 2019-09-11 16:34
This tutorial shows how to create a fade effect in JavaScript without using libraries such as jQuery.
We will be using CSS opacity to dynamically control the opacity of the elements with JavaScript. Some backwards compatibility code for older browsers is also included, it is safe to remove this if not needed.
There is a complete example located at the end of the Tutorial, you may skip this explanation if not needed. Just remember that you can control the different timers in the three variables: duration, hidtime, showtime.
The CSS below should be placed in the head section of the HTML .
#FadeBox {
opacity: 1.0; /* Standard */
/* Older browsers */
-moz-opacity: 1.0; /* Older versions of Firefox */
-khtml-opacity: 1.0; /* Older versions of Safari */
filter: alpha(opacity=100); /* Internet Explorer */
}
The above code is applied the element with the FadeBox ID, but you can name the element whatever you want – just remember to change the ID everywhere it is being used.
At the beginning of the script are some variables to help you control the timers. The duration of the fade effect itself is controlled with the duration variable, while the length of time the element should stay hidden and visible is controlled with the hidtime and showtime variables.
var element = document.getElementById("FadeBox");
var duration = 3000; /* fade duration in millisecond */
var hidtime = 2000; /* time to stay hidden */
var showtime = 2000; /* time to stay visible */
The SetOpa function is used to change the opacity of the element dynamically from within a loop. Again, there is some code used for compatibility with older browsers.
function SetOpa(Opa) {
element.style.opacity = Opa;
<!-- Code for older browsers below -->
element.style.MozOpacity = Opa;
element.style.KhtmlOpacity = Opa;
element.style.filter = 'alpha(opacity=' + (Opa * 100) + ');';
}
Old versions of Internet Explorer does not support opacity, so we are using it's filter instead. The filter uses a number between 0 and 100 rather than 0.0 and 1.0. This is easily solved by Multiplying the Opa value with 100 for the filter version.
The loops in the fadeIn and fadeOut functions are used to set the opacity by calling the SetOpa function inside the loop.
function fadeOut() {
for (i = 0; i <= 1; i += 0.01) {
setTimeout("SetOpa(" + (1 - i) +")", i * duration);
}
setTimeout("fadeIn()", (duration + hidtime));
}
The main part of this function is the for loop. Basically the loop runs as long as the variable i is smaller then 1 (1.0).
The SetTimeout function will perform actions after a duration of time. We use this function to feed our SetOpa function with the current value of i, (in this case i subtracted from 1).
The math
setTimeout("SetOpa(" + (1 - i) +")", i * duration);
i is multiplied by the duration variable, to time and place the SetOpa function calls in a sequence. Removing it to see what happens may help you understand the code better.
Finally we call fadeIn. By adding the fade duration with the hidtime, we can time the fadein to after the hidtime has ended.
setTimeout("fadeIn()", (duration + hidtime));
The full script
<!DOCTYPE html>
<html lang="en-US">
<head>
<title>JavaScript Fade - beamtic Example</title>
<style type="text/css">
#FadeBox {
opacity: 1.0;
-moz-opacity: 1.0;
-khtml-opacity: 1.0;
filter: alpha(opacity=100);
}
</style>
</head>
<body>
<div id="Basement" style="width:90%;margin:auto;">
<h1 onClick="fadeOut()">Fade Example</h1>
<div style="width:100%;" id="FadeBox">
<p>This is a JavaScript Fade example, using setTimeout to change the CSS opacity of elements. This should work on most objects, including images!</p>
<p>You can use this to rotate your news stories, and as such save some precious space. Just be sure to include a "Show all stories" link, to be nice to your users.</p>
</div>
<h2>About this Example</h2>
<p>This example shows how to create a JavaScript fade effect. </p>
<p>If you like this example, be sure to check out the rest of <a href="http://beamtic.com/">beamtic</a>.</p>
</div>
<script type="text/javascript">
var element = document.getElementById("FadeBox");
var duration = 3000; /* fade duration in millisecond */
var hidtime = 2000; /* time to stay hidden */
var showtime = 2000; /* time to stay visible */
function SetOpa(Opa) {
element.style.opacity = Opa;
element.style.MozOpacity = Opa;
element.style.KhtmlOpacity = Opa;
element.style.filter = 'alpha(opacity=' + (Opa * 100) + ');';
}
function fadeOut() {
for (i = 0; i <= 1; i += 0.01) {
setTimeout("SetOpa(" + (1 - i) +")", i * duration);
}
setTimeout("FadeIn()", (duration + hidtime));
}
function FadeIn() {
for (i = 0; i <= 1; i += 0.01) {
setTimeout("SetOpa(" + i +")", i * duration);
}
setTimeout("fadeOut()", (duration + showtime));
}
</script>
</body>
</html>
Tell us what you think: