PHP: Parse_url Function
How to use the parse_url function to obtain different parts of a URL.
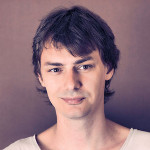
By. Jacob
Edited: 2020-05-30 01:00
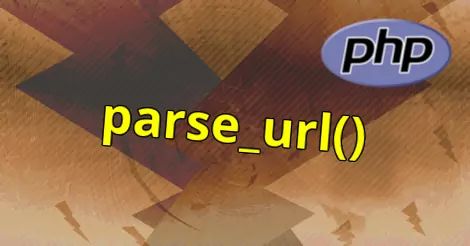
The PHP parse_url function splits up a URL into different parts, and makes it easy to access the part of the URL that is of interest, whether it being the domain name, the requested path, or even the URL parameters.
Normally you will not have to work directly on the bare query string, since you can access individual parameters through the $_GET super global.
To parse any given URL, we may pass the URL to the parse_url function. For example, if we want to parse the full requested URL, we can do so using a combination of the REQUEST_URI and HTTP_HOST variables, which we feed to PHP's parse_url function.
If you want to get a specific part, it can help to store the parsed URL in a variable:
$url_to_parse = 'http://'. $_SERVER['HTTP_HOST'] . $_SERVER['REQUEST_URI'];
$parsed_url = parse_url($url_to_parse);
echo $parsed_url['path'];
Note. If obtaining the exact requested URL is important to you, including the protocol (http or https), then you should read this article: Get The Full Requested URL in PHP
Parsing URLs with parse_url
When you store the parsed URL in a variable, different parts will be made available to you as an associative array.
Note. For a given part to be available, it will have to be present in the URL that you are parsing.
The different parts are as follows:
- scheme
- host
- port
- user
- pass
- path
- query
- fragment
The fragment part is the hash (#) used to link to subsections in HTML pages. It is not available from the server-side via server variables, since it is never sent as part of the HTTP request. Nevertheless, it is still accessible in static URLs.
To show the different available parts for a given URL, you could var_dump the returned associative array:
var_dump(parse_url('http://'. $_SERVER['HTTP_HOST'] . $_SERVER['REQUEST_URI']));
Output:
array(3) {
["scheme"]=> string(4) "http"
["host"]=> string(16) "beamtic.com"
["path"]=> string(17) "/index.php"
["query"]=> string(16) "test=just_a_test"
}
Tell us what you think: