PHP: Associative Arrays
In PHP all arrays can be Associative. In this tutorial, you will learn how to create an association between keys and values in arrays.

Edited: 2021-03-07 10:03
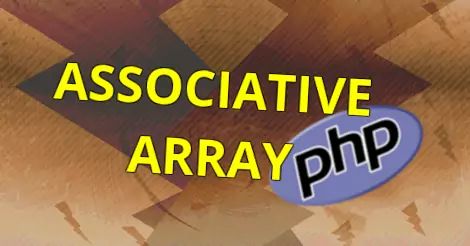
An Associative Array is an array that uses strings as keys; typically there will also be a relationship between the keys and the values in the array.
In PHP, it does not matter if you use integers or strings as keys, since PHP does not distinguish between indexed and associative array types. You could even use a mix of strings and numbers as keys if you wanted.
An Associative arrays is commonly used for mapping values to corresponding keys, which indicates an association between the key and a value pairs in the array; but this usually also means that the key should not be changed, and you should avoid using build-in PHP functions that does not preserve the relationship between keys and values.
Taking a very basic example, using a single-dimensional array to store names and e-mail addresses of some of PHP's creators:
$teachers = array(
'Rasmus Lerdorf' => 'rasmus.l@example.com',
'Zeev Suraski' => 'zeev.s@example.com',
'Andi Gutmans' => 'andi.g@example.com'
);
To store more more information for each teacher, such as the year the teacher was born, we could use a two-dimensional array like this:
$teachers = array(
'Rasmus Lerdorf' => array('e-mail' => 'rasmus.l@example.com', 'born' => '1968'),
'Zeev Suraski' => array('e-mail' => 'zeev.s@example.com', 'born' => '1976'),
'Andi Gutmans' => array('e-mail' => 'andi.g@example.com', 'born' => '1972')
);
Traversing an associative arrays
The foreach loop construct is ideal for iterating over an associative array; when a foreach loop is used, both the key and the value will be made available as variables.
First, this is how to iterate over a single-dimensional array:
foreach ($teachers as $teacher => $email) {
echo PHP_EOL . $teacher . ':' . $email;
}
Output:
Rasmus Lerdorf:rasmus.l@example.com
Zeev Suraski:zeev.s@example.com
Andi Gutmans:andi.g@example.com
To iterate over multi-dimensional arrays can be a challenge, especially if we do not know the level of nesting the array is going to have. However, for a simple two-dimensional array like the one used in this tutorial, you could just "hard-code" the code that deals with the second-level arrays:
foreach ($teachers as $teacher => $data) {
echo PHP_EOL . $teacher . PHP_EOL .
' E-mail: ' . $data['e-mail'] . PHP_EOL .
' Born: ' . $data['born'];
}
Output:
Rasmus Lerdorf
E-mail: rasmus.l@example.com
Born: 1968
Zeev Suraski
E-mail: zeev.s@example.com
Born: 1976
Andi Gutmans
E-mail: andi.g@example.com
Born: 1972
Objects vs arrays
When you are working with object orientated PHP, it is often better to use objects instead of arrays; this is because of benefits such as security added from type hinting and auto completion in your code editor.
Objects can also be iterated over using a foreach loop, so they are almost functionally identical when only used with properties.
If you were to remake the $teachers array from earlier, then you could do it like so:
$teachers = new teachers();
$teachers->{'Rasmus Lerdorf'} = 'rasmus.l@example.com';
$teachers->{'Zeev Suraski'} = 'zeev.s@example.com';
$teachers->{'Andi Gutmans'} = 'andi.g@example.com';
foreach ($teachers as $name => $email) {
echo $name . ": ", $email . '<br>';
}
This example uses complex syntax to create the property names, since normally you would not be able to include spaces in property names; by using the complex curly syntax, we can use any character as the property name, similarly to how we may use any character as a key value in an array.
Auto completion
The above example is not very good, since it might break auto completion because of the use of complex syntax. Instead, you should consider the below example:
class student {
public $name;
public $age;
public function __construct(string $name, int $age)
{
$this->age = $age;
$this->name = $name;
}
}
$some_student = new student('Jacob', 20);
echo $some_student->name;
This allows you to leverage auto-completion in an editor like Visual Studio Code with PHP Intelephense:
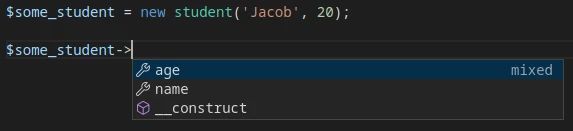
To iterate over the object, you can still use a foreach loop:
foreach ($some_student as $key => $value) {
echo $key . ": ", $value . '<br>';
}
Type hinting
Any named class can be used as a type in PHP, this means we can use the student class as a type in the class definitions of objects that might depend on it.
The benefit from this is that PHP will throw an error if an object of the wrong type is passed to the class, which helps developers catch errors early. Below is a full example of how this works — you can try passing on an incorrect object to see what happens.
class student
{
public $name;
public $age;
public function __construct(string $name, int $age)
{
$this->age = $age;
$this->name = $name;
}
}
class teacher
{
private $student;
public function __construct(student $student)
{
$this->student = $student;
echo '<p>The name of the student I am teaching today is: </p>';
echo "<p><b>{$this->student->name}</b></p>";
exit();
}
}
Tell us what you think: