PHP: Stop Code Execution with Exit
How we can use the PHP's exit function to stop the execution of remaining code.
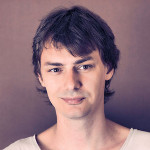
By. Jacob
Edited: 2021-02-07 12:20
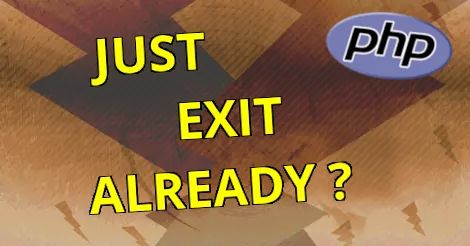
exit is usually used right after sending final output to a browser, or to stop a script in case there was an error. When exit is used it will stop code-execution completely and exit PHP.
You should use return when you want to send a value back to the calling location, and exit when you want PHP to stop executing the rest of your script. Here is an example of how to exit a script:
echo '<p>Hallo World</p>';
exit();
// Code past this point will not be executed
// ... more code here
exit is often used for quick and dirty bug hunting by developers when they want to test output; when this is done, output is usually sent with echo, print, print_r, and var_dump It is probably quite common to have a function to output the content of variables:
function dumpText($variable) {
header('content-type: text/plain; charset=utf-8');
var_dump($variable);
exit();
}
Another common place to call exit is after sending a location header redirect:
header('Location: https://beamtic.com/');
exit();
Calling exit from functions and methods
When a function or method is called, it will usually return to the calling script once it finishes executing the relevant function-code.
If for example we responding to a HTTP request from within a function, it would often be desired to have the script stop executing any remaining code. I.e.:
function respond_not_found($code) {
http_response_code(404);
header('Content-Type: text/html; charset=UTF-8');
echo '<h1>404 Not Found</h1>' .
'<p>Eh!? Now you are just guessing...</p>';
exit();
}
Then, when checking the requested page, we can simply output a 404 and exit when the page is not found:
$valid_system_pages_arr = array('frontpage' => true, 'categories' => true, 'blog' => true);
if (isset($valid_system_pages_arr["$requested_page"]) == false) {
respond_not_found();
}
// The rest of our code goes here :-D
echo 'A valid system page was requested.';
Exiting from within loops
We can not use exit inside a loop, since it would completely stop running the rest of the program. Usually, you would want to just stop executing the rest of the loop, for this we can use the break keyword.
However, using the break keyword would usually rise the need to use conditional logic inside of the loop, which can be inefficient, depending on the circumstances.
Using the break keyword to stop a loop from running:
$i = '1';
while ($number <= '15') {
break(); // Only the loop stops executing
echo 'Run Number:' . $number . "\n";
++$number;
}
The below would not work:
$i = '1';
while ($number <= '15') {
exit(); // The program exits on the first run without running any remaining code
echo 'Run Number:' . $number . "\n";
++$number;
}
// This code will not be executed, since we "exit" the script inside the loop
Variable-dumping
Variable-dumping works well for learning the structure of a variable's contents, this is also commonly done when debugging and bug hunting — but as an application grows you will probably want more sophisticated error handling.
Often, when PHP developers are debugging applications, they will be using var_dump and print_r in combination with exit to output the content of variables and objects. This can be practiced from anywhere within a script, even from methods in a Object Orientated application.
An example of this would be:
$my_array = array('bug', 'hunt', 'professionals');
$some_variable = 'test';
var_dump($some_variable);
print_r($my_array);
exit();
It is easier to have a function declared somewhere in the global scope:
function dumpHTML($variable) {
header('content-type: text/html; charset=utf-8');
var_dump($variable);
exit();
}
Tell us what you think: