Nested Functions in PHP
About using nested functions in PHP (functions inside of functions).
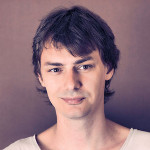
By. Jacob
Edited: 2021-02-05 22:40
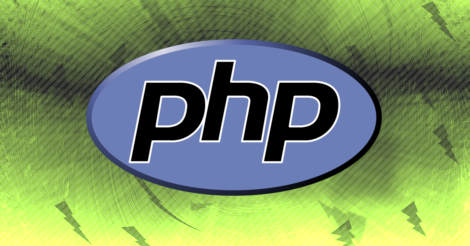
Nested functions (Aka: functions inside functions) are possible in PHP, and sometimes used in the form of anonymous functions.
It is also possible to create named functions inside of other functions, just as you do in procedural PHP; but I would not recommend this. One place where this might be useful is for polyfills, though I have not seen practical examples of it.
The problem with nested functions is that they are re-declared every time a function is called; which means that they come at a small performance hit. They also pollute the global scope, since they become available globally once declared; because of this, you may instead want to learn about Object Orientated PHP.
A nested function in procedural PHP looks like this:
function my_parent_function() {
say_hallo();
function say_hallo() {
echo 'Hallo World!';
exit();
}
}
// Call the parent function
my_parent_function();
Nested functions in PHP
When a function is defined inside a parent function, you will first have to call the parent function before the child function becomes available. Once the parent has been called, child functions will be available globally in your PHP script, and not just in the parent.
If you still want to get your hands dirty with nested functions, you should check out the below example:
function MyFunc() {
// The Nested Functions
function DoThis() {
return 'Yeah!';
}
function DoThat() {
return 'Nah!';
}
}
// Calling MyFunc will define the child-functions
MyFunc();
echo DoThis();
You may also use the nested functions inside of the parent:
function MyFunc($content) {
// The Nested Functions
function DoThis() {
return 'Yeah!';
}
function DoThat() {
return 'Nah!';
}
// The Main Function Script
if ($content == 'Yes yes') {
return DoThis();
} else {
return DoThat();
}
}
// The Main Script
$string = 'Yes yes';
echo MyFunc($string);
Anonymous functions
When you use an anonymous function inside a function or method it is also a type of nested function. The syntax of anonymous functions in PHP is similar to the syntax used in JavaScript.
Anonymous functions are useful for many purposes, one is when you use the array_walk function to "walk over" an array:
$my_array = ['PHP7', 'Scripting']
array_output($my_array);
function array_output($array) {
array_walk($array, function ($value, $key) {
echo PHP_EOL . $key . ':' . $value;
});
}
Object-oriented PHP
Using an objected orientated approach may be more approiate, and will also give more control over where the methods (functions) are accessible from.
For example, when defining functions (methods) inside of classes in PHP, it is possible to define them using the private and public keywords.
Defining a method as private will only make it usable from within the class it belong to. If the method is defined using public instead, it will be made accessible even outside of the class using $MyObject->PublicFunction(); which is useful when writing more maintainable and portable code.
Links
- User-defined functions - php.net
Tell us what you think:
I think this is the way with no side effect, am I right?
function main(){
$a = function(){
echo 'a';
};
}
main();
$a(); // throws error
Within a function, you can define a variable to be a function. Variables are local.
function MyFunction($y)
{
$mysubfunction = function($x)
{
return $x + 10;
};
$y = $mysubfunction($y);
echo $y;
}
MyFunction(12);