PHP: Type Hinting and Default Values
Default values have a tendency to make PHP choke up when using type hinting, but there is a way around the issue.
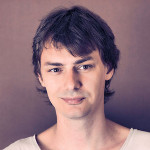
By. Jacob
Edited: 2020-05-30 00:39
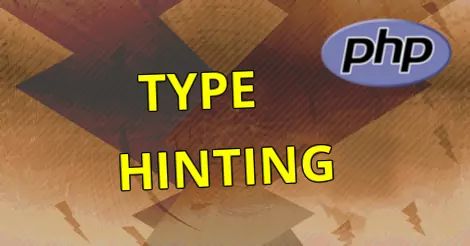
When using type hinting in PHP 7 together with default values on function and method arguments, you have probably noticed that you can not have a boolean default value, such as true or false, while also accepting a string as input — you can, however, use null as a default value.
There is at least a couple of solutions to this problem. First, you may consider simply using a default value of the same type as the input. This is the easiest solution, and there is probably rarely a functional difference.
I find that using a boolean as a default value is useful when a function argument is not used, but it is hard while maintaining the luxury of type hinting.
The second solution is to to use an implementation of Named Parameters, complete with default values and type checking.
The final solution would be to define a default within the function itself:
function welcome(string $name = '') : string {
if ($name == '')) {
$name = 'Anonymous';
}
return 'Welcome ' . $name;
}
echo welcome();
Or, even shorter:
function welcome(string $name = '') : string {
$name = ($name == '') ? 'Anonymous' : $name;
return 'Welcome ' . $name;
}
echo welcome();
Using null as a default value
If a parameter is not required, you may use null as a default value. Checking for null is not any more difficult than checking for a boolean value.
Using an if statement we can easily check for null like this:
function that_is(string $some_input = null) {
if(null === $some_input) {
echo 'hallo';
} else {
echo $some_input;
}
}
Note. When the triple equal sign ===, also known as the identical operator, both the data type and content is compared.
This will only work with null, so we will not be able to string or integer as a default value, for example.
Tell us what you think: