If, Else if, Else in PHP
Learn how to write if statements in PHP in this tutorial. It also includes a video for those who prefer to get things explained.
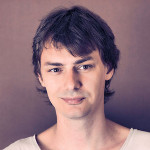
By. Jacob
Edited: 2021-02-21 15:01
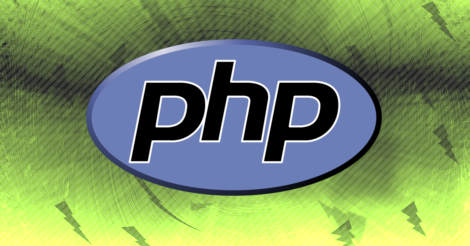
If statements are conditional statements, which allows execution of fragments of code, all depending on what condition has been meet.
Basic if statements's are useful to check for conditions, such as, whether a user is logged in. You can also include so called else if's in statements, which allows you to run alternative code, in case the condition that you have set wasn't meet.
If, else
In the following example, we will be using the PHP rand function to create a "random" number between 1 and 2, which we can use to compare with a static value in our if statement.
If the number is 1, we will echo "The number was 1", and accordingly "The number was 2" if the number is determined to be "2".
$RandomNumber = rand(1, 2);
if ($RandomNumber == '1') { // PHP If Statement
echo 'The number was 1';
} else { // PHP Else
echo 'The number was 2';
}
If the number was not found to be "1", we simply assume it was "2". Assumptions like these are often safe to make, and it may be better than doing multiple if statements.
Else if
The else if may be used to check for additional conditions. Use the same approach as before, but this time use 4 numbers instead.
Keep in mind, there is no need to perform a check when you can predict the outcome. So, we simply include a normal "else" at the end instead of performing another check.
The "else" at the end will be used to execute the the code for when the number is "4".
$RandomNumber = rand(1, 4);
if ($RandomNumber == '1') { // PHP If Statement
echo 'The number was 1';
} elseif ($RandomNumber == '2') { // PHP Elseif Statement
echo 'The number was 2';
} elseif ($RandomNumber == '3') { // PHP Elseif Statement
echo 'The number was 3';
} else {
echo 'The number was 4'; // PHP Else
}
Multiple checks in one statement
You can combine multiple if checks into one statement by using the "&" operator, and as such, you do not need to write a statement for each and every check you will be doing. Instead you can opt to perform multiple checks in one go, like this:
$Number = 5;
$Letter = 'T';
if (($Number == '5')
&& ($Letter == 'T')) { // PHP If Statement with multiple checks
echo 'Both the Number and the Letter matched!';
} else { // PHP Else
echo 'Something did not match!';
}
Adding a third check to the same statement, would simply be done like below:
$Number = 5;
$Letter = 'T';
$Word = 'Beamtic';
if (($Number == '5')
&& ($Letter == 'T')
&& ($Word == 'Beamtic')) { // PHP If Statement with multiple Checks
echo 'Both the Number, Letter and Word matched!';
} else { // PHP Else
echo 'Something did not match!';
}
Abbreviated statements
Abbreviated If statements is a shortened form, which is leaving out the curly brackets ({}).
These statements are useful when you only have a single line of code to be executed, allowing you to leave out the curly brackets, for convenience. It can however quickly course problems if you forget it later, and then change the statement, so we do not recommend doing this.
if ($RandomNumber == '1')
echo 'The number was 1';
echo 'More stuff..'; // will be executed regardless of the check.
Another Example, including an else.
if ($RandomNumber == '1')
echo 'The number was 1';
else
echo 'The number was not 1';
Using colon instead of curly brackets
You can also use colon instead of curly brackets, which to use may be mostly down to personal preference. We recommend to use the brackets, and not to use this method. If you decide to use it anyway, this is how it may be done:
if ($RandomNumber == '1'):
echo 'The number was 1';
elseif ($RandomNumber == '2'):
echo 'The number was 2';
elseif ($RandomNumber == '3'):
echo 'The number was 3';
else:
echo 'The number was 4';
endif;
Operators
The below can be used to make comparisons like, less then, greater then, and not equal to:
< | Less then |
> | Greater then |
= | Assignment operator |
== | Equal to |
=== | Equal to and of same type |
!= | Not Equal to |
<= | Less then or Equal to |
>= | Greater then or Equal to |
Note. These operators are not exclusive to if statements.
The difference between double equals signs (==), and a single equals sign (=), is that when you are using a single equals sign in a if statement, you will be assigning a value to a variable while checking if the assignment was successful, rather than comparing it. So, always use at least two equals signs when comparing.
Further more, when you are comparing numbers, you should consider using three (===) equals signs to avoid mistaking the boolean values true and false with the numeric 1 and 0. Alternatively you may also use greater than (>), and less than (<) to avoid the problem. This situation is tested at 4:03 in the video.
Tell us what you think: