What is Ajax?
AJAX is simply a way to perform HTTP requests in JavaScript.
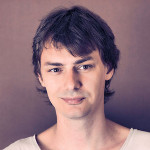
By. Jacob
Edited: 2019-10-13 05:39
Ajax stands for Asynchronous JavaScript and XML, and is simply a technique in JavaScript used to create websites or web applications, that loads- and sends data asynchronously from- and to the server.
AJAX can be used to send HTTP requests to the server silently in the background. The server typically responds to these requests by ending a 200 ok response code together with a response body.
The body part of the response is often just plain HTML or XML, which can then be inserted directly via JavaScripts innerHTML property. However, many modern applications now use JSON object as the response. JSON objects can easily be concerted to arrays using build-in functions in JavaScript.
httpClient = new http_client();
httpClient.get("/request_handler.php", callback);
function callback(response) {
// Do stuff here
alert(response);
}
Asynchronous JavaScript
Asynchronous simply means that a function will not block the execution of the rest of your script. If you have a background in PHP, then this can be extremely confusing at first since there is nothing indicating why your HTTP requests is not returning anything.
The problem is, you will have some code further down your script that relies on the result of the HTTP request being returned, but since the function is non-blocking, the rest of your script will be executed before your HTTP request function returns. One way to solve this, rather than fighting it, is to accept the asynchronous nature of the function.
To solve this problem, we may use a "callback" function. A callback function is simply a function you call when the result of the HTTP request has been returned or downloaded. Note, however, you will need to continue working within your callback function rather than returning to your composition root. This may look like this:
httpClient = new http_client();
httpClient.get("/request_handler.php", callback);
function callback(response) {
// Do stuff here
alert(response);
}
It took me a long time to figure this out. So, the way we would do it in PHP is actually wrong in JavaScript:
// "Wrong" way to do it
httpClient = new http_client();
response = httpClient.get("/request_handler.php");
alert(response);
This is called callback hell by some.
The above example is using Beamtic's HTTP Client, which is a JavaScript class for sending HTTP requests. It is good practice to write your own AJAX functions in pure JavaScript, but in production it is usually better to use a library or class you include, as it might increase compatibility with browsers.
Tell us what you think: