AutoIt MouseClick Function
Automating mouse clicks with the MauseClick function.

Edited: 2021-02-14 02:21
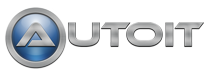
The AutoIt MouseClick function is used to automate the mouse and send mouse clicks. The way it works is by moving the mouse to the given screen coordinates, at a given speed, and then perform the number of clicks asked of it, with the given mouse button.
There is a tool that comes with AutoIt called Window Info which can be used to get the coordinates of the screen.
If the AutoIt Window Info tool is used to get the screen coordinates which are to be clicked, the coord mode setting of the tool will influence the returned pixel coords. This can sometimes cause scripts to click in unexpected coordinates. To avoid this, be sure to set the MouseCoordMode option in your scripts so that it matches the Coord Mode setting in the Window Info tool. I.e.:
Opt("MouseCoordMode", 1) ; Sets the coord mode to use absolute screen coordinates
MouseClick("primary", 200, 150, 50)
The coord mode values are as follows:
0 | Coordinates relative to the active window |
1 | Absolute screen coordinates (default) |
2 | Coordinates relative to the client area of the active window |
Parameters
The MouseClick function accepts 4 Parameters, the first the button to click with, the second and third is the pixel based left and right position on the screen, while the final value to be given is the movement speed of the mouse.
First parameter:
left
right
middle
main
menu
primary
secondary
Note that primary is used to refer to the primary mouse button, this is useful since a user might change their key mappings — same logic applies for the secondary value.
2nd parameter:
The (x) position from the top in pixels.
3nd parameter:
The (y) position from the top in pixels.
4nd parameter:
The movement speed of the mouse, takes a value from 1 to 100.
Examples
The first example only uses the MouseClick function to perform a one time click on the supplied screen coordinates. If the pointer is not already at the location, the pointer will automatically move there with a speed of 50 (the last function parameter).
MouseClick("primary", 200, 150, 50)
In the second example, the script will repeatedly click the same spot on a screen until an exit hotkey is pressed. To accomplish this, we can place the MouseClick function inside a while loop like so:
;Assigns the HotKey to our custom exit function
HotKeySet("^!x", "MyExit")
; The coordinates to click in pixels
$x = 200
$y = 150
$i = 0
While $i <= 10
MouseClick("left", $x, $y, 1)
; Uncomment the below line to make the script click only 10 times, or until the hotkey is pressed.
; $i = $i + 1
; Uncomment the below line to make the script pause between clicks, the value is in milliseconds
; Sleep(5000)
WEnd
Func MyExit()
Exit
EndFunc
The above script consists of two important parts, the first being the Infinite loop, which can only be stopped with the hotkey that we set in the beginning of the script using the HotKeySet function. The second part is the function at the end of the script, defined using the Func statement, which we bound to our hotkey at the beginning of the script. See also: User functions
Tell us what you think: