GET, POST, and HEAD requests with cURL in PHP
How to use cURL to send HTTP GET and POST requests from PHP applications.
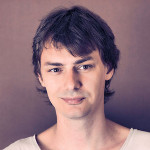
By. Jacob
Edited: 2021-04-12 08:44
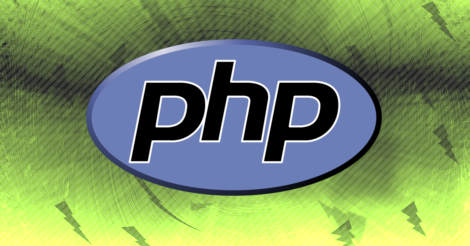
In addition to allowing us to send HTTP requests through the file- functions, PHP also has a library called cURL for sending HTTP requests; both methods enables you to do the same things, but cURL may be easier to use than stream_get_contents.
You will often be using cURL when you can not use the PHP file- functions; and when cURL is not installed on a server, you may try to use the file- functions in combination with stream_get_contents instead. Which one you use is not really important, but some will find that cURL is more easy to use.
Often you will just need to perform a GET request for a resource in order to download it, and work with the content locally; to do this, you may use the following code:
// URL to fetch
$url = "https://beamtic.com/api/user-agent";
// Initialize cURL session
$ch = curl_init($url);
// Option to Return the Result, rather than just true/false
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Perform the request, and save content to $result
$result = curl_exec($ch);
// Close the cURL resource, and free up system resources!
curl_close($ch);
// Shows the result!
echo $result;
cURL GET request
Sending a GET request with cURL is done using a combination of curl_init, curl_setopt, curl_exec, and curl_close.
curl_init initializes a cURL session, in this script, referenced in via the $ch variable. The curl_setopt function can be used to set different options for our session, while curl_exec is used to send the request.
// URL to fetch
$url = "https://beamtic.com/api/user-agent";
// Initialize cURL session
$ch = curl_init($url);
// Option to Return the Result, rather than just true/false
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Perform the request, and save content to $result
$result = curl_exec($ch);
// Close the cURL resource, and free up system resources!
curl_close($ch);
// Shows the result!
echo $result;
The curl_close function is used to close the session. Generally, PHP will clean up when a script finishes running, so it is not really needed.
Sending a GET with a message body
It is also possible to send a GET request with a payload. This may be useful when communicating with various APIs on the internet. In order to do this, you just need to include the CURLOPT_POSTFIELDS option in your preparation of the request:
$ch = curl_init("https://beamtic.com/api/user-agent");
$payload = '{}';
curl_setopt($ch, CURLOPT_POSTFIELDS, $payload);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Perform the request, and save content to $result
$result = curl_exec($ch);
// Shows the result!
echo $result;
Sending a message body with a GET is valid according to the the specification.
Read: rfc2616#section-4.3
Responses to a HEAD request can not contain a message body.
The CURLOPT_POSTFIELDS option is also used when sending POST requests.
cURL POST request
When sending a POST request with cURL, you should beware that providing the post fields in an array is likely to cause problems in some cases, as it will cause cURL to send the request as multipart/form-data with a boundary, instead of sending it as application/x-www-form-urlencoded – this is rather unexpected behavior.
We need to do two things to send a post request, set the CURLOPT_POST option to true, and include the post fields via the CURLOPT_POSTFIELDS option. Both can be set using the curl_setopt function, before executing the request.
A simple POST request is performed as follows:
$url = "http://beamtic.com/Examples/http-post.php"; // The POST URL
// The POST Data
$postdata = "name=Jacob";
$postdata .= "&bench=150";
$ch = curl_init($url);
// Set the request type to POST
curl_setopt($ch, CURLOPT_POST, true);
// Pass the post parameters as a naked string
curl_setopt($ch, CURLOPT_POSTFIELDS, $postdata);
// Option to Return the Result, rather than just true/false
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Perform the request, and save content to $result
$result = curl_exec($ch);
// Show the result?
echo $result;
The important part in the above script is the part where you set the request-type to POST, and pass the data to be posted:
// Set the request type to POST
curl_setopt($ch, CURLOPT_POST, true);
// Pass the post parameters as a naked string
curl_setopt($ch, CURLOPT_POSTFIELDS, $postdata);
The variable $postdata contains a string where each parameter is separated by unencoded ampersands. To easily add and remove parameters in a very read-able way, you can do like this:
// The POST Data
$postdata = "name=Jacob";
$postdata .= "&bench=150";
This is just as clean and readable as using an array.
Sometimes the data in some post fields contains special characters, these will need to be encoded with PHP's urlencode function. The server handling the request should automatically decode them, without the need for extra coding.
$postdata = "name=" . urlencode($userinput);
$postdata .= "&bench=150";
HEAD Request
A HEAD request may be sent by using a combination of cURL options.
1. The CURLOPT_NOBODY option needs to be set to true
2. To have the response returned to you, the CURLOPT_RETURNTRANSFER should also be set to true
3. CURLOPT_HEADER should be true to include the headers in the output.
Example follows:
$url = 'https://beamtic.com/';
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_NOBODY, true);
curl_setopt($ch, CURLOPT_HEADER, true);
$response = curl_exec($ch);
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
exit();
}
curl_close($ch);
// Output the response:
echo $response;
To perform a similar HEAD request from a terminal you can do like this:
curl --HEAD http://beamtic.com/
For more details, and information about parsing HTTP response headers you should read:
Installing PHP-cURL
The cURL library is commonly used and often installed by default, so you should not have to do anything yourself before being able to use it. However, people who work locally or manage their own servers might need to install it. How to do this will depend on the system.
If cURL is not installed you may get an error like this:
PHP Fatal error: Call to undefined function curl_init() in my_http_client.php on line 15
On Debian and Ubuntu you will just need to type a command in the terminal to install the library; but note that the package-version should correspond to the version of PHP you got installed on your system. To install the cURL library, you may type something like this:
sudo apt install php8.0-curl
If you are not sure what version of PHP you got installed, you can try typing php -v in a terminal, this should return something like:
PHP 8.0.1 (cli) (built: Jan 13 2021 08:22:35) ( NTS )
Copyright (c) The PHP Group
Zend Engine v4.0.1, Copyright (c) Zend Technologies
with Zend OPcache v8.0.1, Copyright (c), by Zend Technologies
Alternatively, you can also put phpinfo(); inside a PHP script and try opening it in your browser.
Finally, if you are having trouble finding the correct package to install, you can search for it from the comfort of your terminal:
sudo apt-cache search php
Or, if you know that the extension you need to install is for PHP8:
sudo apt-cache search php8
This will result in something like:
libapache2-mod-php8.0 - server-side, HTML-embedded scripting language (Apache 2 module)
libphp8.0-embed - HTML-embedded scripting language (Embedded SAPI library)
php8.0 - server-side, HTML-embedded scripting language (metapackage)
php8.0-amqp - AMQP extension for PHP
php8.0-apcu - APC User Cache for PHP
php8.0-ast - AST extension for PHP 7
php8.0-bcmath - Bcmath module for PHP
php8.0-bz2 - bzip2 module for PHP
php8.0-cgi - server-side, HTML-embedded scripting language (CGI binary)
php8.0-cli - command-line interpreter for the PHP scripting language
php8.0-common - documentation, examples and common module for PHP
php8.0-curl - CURL module for PHP
php8.0-dba - DBA module for PHP
php8.0-dev - Files for PHP8.0 module development
This returns a list of packages that you can install. Note the lines that appear like php8.0-* are often extensions for PHP; of course the version should correspond with the version of PHP you got installed.
Tools:
You can use the following API endpoints for testing purposes:
https://beamtic.com/api/user-agent
https://beamtic.com/api/request-headers
Tell us what you think: