Using proxy servers with PHP's build-in HTTP functions
How to perform HTTP requests trough proxy servers in PHP.
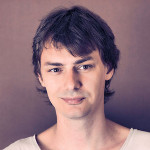
By. Jacob
Edited: 2021-04-12 08:45
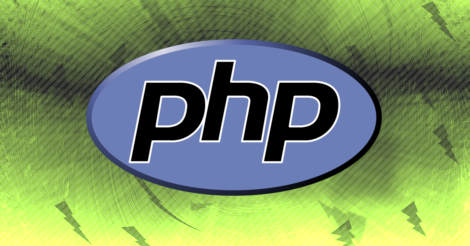
Sometimes, for whatever reason, you may need to hide your own IP address. A common way to hid your IP is to use a proxy server to process your HTTP requests. Setting up your browser to use a proxy server is easy enough, but setting up scripts and programs to use proxies can require a bit more effort. This tutorial shows you how to make your PHP scripts use a proxy when performing HTTP requests with functions such as fread and file_get_contents.
If you remember the example from the last tutorial, adding a proxy can be done as easily as demonstrated in the below example:
$sURL = "http://beamtic.com/Examples/ip.php"; // The Request URL
$aHTTP['http']['proxy'] = 'tcp://127.0.0.1:8118'; // The proxy ip and port number
$aHTTP['http']['request_fulluri'] = true; // use the full URI in the Request. I.e. http://beamtic.com/Examples/ip.php
$aHTTP['http']['method'] = 'GET';
$aHTTP['http']['header'] = "User-Agent: My PHP Script\r\n";
$aHTTP['http']['header'] .= "Referer: http://beamtic.com/\r\n";
$context = stream_context_create($aHTTP);
$contents = file_get_contents($sURL, false, $context);
echo 'Real IP:' . $_SERVER['REMOTE_ADDR'] . '<hr>';
echo 'Proxy IP:'. $contents;
As in the prior Tutorials, you can also use the URL provided in this example for testing purposes. ip.php is a simple php script outputting the IP of the client. If this is different than your own IP, the proxy works.
Proxy Authentication
If the proxy that you are using requires authentication, you will simply add the proxy authorization http header, in a manner similar to what is shown in the last php tutorial. Logging in to a proxy is done by combining the username and password, only separated by a single colon ":", then encoding it using base64, and send it in the Proxy-Authorization http header like shown in below example:
$sLogin = base64_encode('username:password');
$sURL = "http://beamtic.com/Examples/ip.php"; // The Request URL
$aHTTP['http']['proxy'] = 'tcp://127.0.0.1:8118'; // The proxy ip and port number
$aHTTP['http']['request_fulluri'] = true; // Use the full URI in the Request. I.e. http://beamtic.com/Examples/ip.php
$aHTTP['http']['method'] = 'GET';
$aHTTP['http']['header'] = "User-Agent: My PHP Script\r\n";
$aHTTP['http']['header'] .= "Referer: http://beamtic.com/\r\n";
$aHTTP['http']['header'] .= "Proxy-Authorization: Basic $sLogin";
$context = stream_context_create($aHTTP);
$contents = file_get_contents($sURL, false, $context);
echo 'Real IP:' . $_SERVER['REMOTE_ADDR'] . '<hr>';
echo 'Proxy IP:'. $contents;
Tools:
You can use the following API endpoints for testing purposes:
https://beamtic.com/api/user-agent
https://beamtic.com/api/request-headers
Tell us what you think: