GET, POST, and HEAD requests with PHP's build-in functions
How to send HTTP requests using the build-in file functions of PHP.
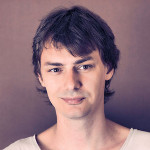
By. Jacob
Edited: 2021-04-12 08:46
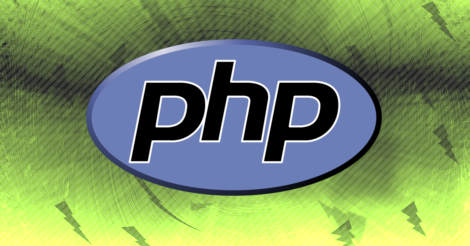
There are a few ways to perform HTTP requests in PHP, in this tutorial we will show how to send a POST and GET request by using the file- functions in combination with stream_context_create.
While using a library like cURL is probably one of the most popular ways to perform HTTP requests, you can also use functions such as file_get_contents and fopen.
While the name of these functions do not exactly indicate that they can also be used for HTTP requests, they do actually work quite well for this, and they are also fairly easy to use.
The stream_context_create function mentioned before is used to gain finer control over various options related to a request.
There should be no disadvantage to using the build-in functions instead of cURL, and unlike what is often claimed, they can be used for both POST and GET requests. However, some hosting companies might disable allow_url_fopen, which will break scripts relying on these methods.
GET Requests
To perform a simple get request, we will first create a $handle variable. We can use this to reference the request when storing the downloaded data in the $contents variable using stream_get_contents.
$handle = fopen("https://beamtic.com/", "rb");
$contents = stream_get_contents($handle);
fclose($handle);
The stream_get_contents function automatically downloads a file or web page, however, if you wish to do it manually, for finer control over the process, you may use a while loop in combination with fread:
$handle = fopen("https://beamtic.com/", "rb");
$contents = '';
while (!feof($handle)) {
$contents .= fread($handle, 8192);
}
fclose($handle);
echo $contents;
In this case the last argument in the fread function is equal to the chunk size, this is usually not larger than 8192 (8*1024). Keep in mind that this can be larger or smaller, but may also be limited by the system PHP is running on. You can optimize your PHP scripts by not using a larger chunk-size than that of your storage device.
One of the simplest ways to download a file, is to use file_get_contents. It requires far less code than using the other methods, but it also offers less control over the process.
$homepage = file_get_contents('https://beamtic.com/');
echo $homepage;
POST Requests
Sending a POST request is not much harder than sending GET. We just have to use the stream_context_create function to add the necessary headers to perform the request.
Again, we can do this both with file_get_contents and fopen; but let us just use file_get_contents for now:
$sURL = "https://beamtic.com/Examples/http-post.php"; // The POST URL
$sPD = "name=Jacob&bench=150"; // The POST Data
$aHTTP = array(
'http' => // The wrapper to be used
array(
'method' => 'POST', // Request Method
// Request Headers Below
'header' => 'Content-type: application/x-www-form-urlencoded',
'content' => $sPD
)
);
$context = stream_context_create($aHTTP);
$contents = file_get_contents($sURL, false, $context);
echo $contents;
The $sURL variable contains the POST URL.
The $sPD variable contains the data you want to post. Formatting should match the content-type header.
The $aHTTP array has all of the options, including headers, which will be passed on to stream_context_create.
You can also perform POST requests with the fread function.
$sURL = "http://beamtic.com/Examples/http-post.php"; // The POST URL
$sPD = "name=Jacob&bench=150"; // The POST Data
$aHTTP = array(
'http' => // The wrapper to be used
array(
'method' => 'POST', // Request Method
// Request Headers Below
'header' => 'Content-type: application/x-www-form-urlencoded',
'content' => $sPD
)
);
$context = stream_context_create($aHTTP);
$handle = fopen($sURL, 'r', false, $context);
$contents = '';
while (!feof($handle)) {
$contents .= fread($handle, 8192);
}
fclose($handle);
echo $contents;
Request Headers
If you got multiple headers, remember to separate them with \r\n
The content-type header tells the server how the posted data is formatted. The application/x-www-form-urlencoded content-type is often used by web applications, but you can also encounter multipart/form-data. If an application does not support the content-type you specify, the POST will usually just fail.
The content header contains the actual data that you want to post. This should be formatted in accordance with the content-type you are using.
Alternatively, you may also add options and headers as shown below, this time adding a referer header:
$aHTTP['http']['method'] = 'GET';
$aHTTP['http']['header'] = "User-Agent: My PHP Script\r\n";
$aHTTP['http']['header'] .= "Referer: https://beamtic.com/\r\n";
Additional request headers will be added in the same way; do this by adding a single Carriage return (\r), and a line feed (\n) at the end of each header, followed by whatever header you want to add. Once you have added all the headers, you should end up with something like the below (full script):
$sURL = "https://beamtic.com/api/request-headers"; // The POST URL
$aHTTP['http']['method'] = 'GET';
$aHTTP['http']['header'] = "User-Agent: My PHP Script\r\n";
$aHTTP['http']['header'] .= "Referer: https://beamtic.com/\r\n";
$context = stream_context_create($aHTTP);
$contents = file_get_contents($sURL, false, $context);
echo $contents;
Tools:
You can use the following API endpoints for testing purposes:
https://beamtic.com/api/user-agent
https://beamtic.com/api/request-headers
Tell us what you think:
Thank you so much for this! This is my first time working with objects. I ended up using this to get an HTTP POST command working to interact with Apache Solr.