Track Execution Time in PHP
Performing benchmark testing in PHP by tracking the execution time of pieces of code.
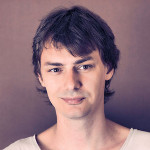
By. Jacob
Edited: 2021-04-27 17:58
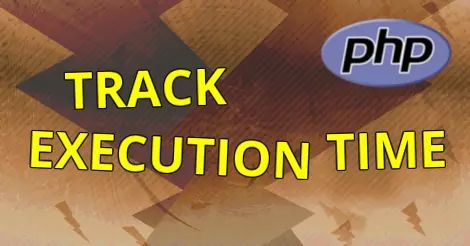
To track the execution time of a PHP script—or just a part of some PHP code—we should make a timestamp right before executing the relevant code, and then again right after the code finishes.
This is quite useful for performing simple benchmark testing of code. Often we will find that there is multiple ways to do the same thing when programming, and this is also true when we are coding in PHP. Some PHP functions are simply faster than others.
For the most part, the speed difference is not going to matter unless you are handling several thousands—or even millions—of requests. But, if we know something is faster, then why not use the most efficient method from the beginning? Very often, it is actually obvious what will be the fastest way.
A simple speed test can be performed like this:
$start_time = microtime(true);
// Your code here
$time_spent = microtime(true)-$start_time;
echo $time_spent;
When the parameter of microtime is set to true, the result will be returned in seconds. If you leave out the parameter, a string will be returned instead of a float, which can not be used easily in calculations; hence why microtime(true) is used instead of clean microtime().
Determining which is faster
In many cases, a single run is not to be enough for us to tell which piece of code is the fastest, since the difference is minuscule.
So, in order to notice a difference, we should combine this with a while or for loop, and then run the test at least a million times:
$start_time = microtime(true);
for ($i = 0; $x <= 1000000; $i++) {
// Your code here
}
$time_spent = microtime(true)-$start_time;
echo $time_spent;
Using the time command in Linux
There is also a command called time available on Linux systems that can be used to exemine the execution time of programs and scripts run from a terminal; to use the command you simply place it in front of whatever command and arguments that you wish to run:
time php name_of_script.php
This should output the total time elapsed while executing a given program:
real 0m0.418s
user 0m0.104s
sys 0m0.314s
The real value represents the total execution time.
Tell us what you think: