Sleep for milliseconds in PHP
How to make PHP sleep for milliseconds rather than seconds.
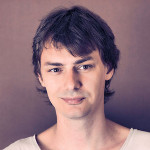
By. Jacob
Edited: 2021-03-07 17:25
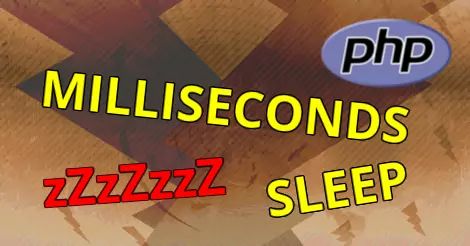
There is no native PHP function to sleep for milliseconds, and you also can not use fractional values in the sleep function, so you will instead need to create your own function; you can do that using usleep, which is a build-in function in PHP used to sleep for microseconds.
Note. A microsecond is one millionth of a second.
To arrive at the milliseconds you multiply with 1000:
function m_sleep($milliseconds) {
return usleep($milliseconds * 1000); // Microseconds->milliseconds
}
Multiplying by another 1000 will get you to seconds:
return usleep($seconds * 1000 * 1000); // Microseconds->milliseconds->seconds
Not that this is needed, since you already got the sleep() function for sleeping for seconds — so try staying with milliseconds for now.
To make things easier, you can create a function that you can call as needed:
m_sleep(5000); // 5000 milliseconds = 5 seconds
function m_sleep($milliseconds) {
return usleep($milliseconds * 1000); // Microseconds->milliseconds
}
Sleep for half a second
You can use the function from before to make a script sleep for half a second. Knowing that a thousand milliseconds is one second, logically, a half second is 0.5 times this value — five hundred; knowing this, you may call the function from before with a value of 500:
echo "Start\n\n";
m_sleep(500); // 500 milliseconds = 0.5 seconds
echo "0.5 seconds has now passed. Exiting.";
exit();
Time conversion table
I thought you might find this table useful, since not everyone remembers what the english definitions corresponds to in raw numbers – and who blames you? It is crazy nuts, off your chair, this stuff!
To one second | |
---|---|
Nanoseconds | 1000000000 (One Billion) |
Microseconds | 1000000 (One Million) |
Milliseconds | 1000 (One Thousand) |
Links
- uslep - php.net
Tell us what you think: