Delayed execution with sleep in PHP
How to delay execution of a PHP script for a specified amount of time.
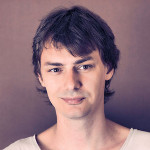
By. Jacob
Edited: 2021-03-07 17:20
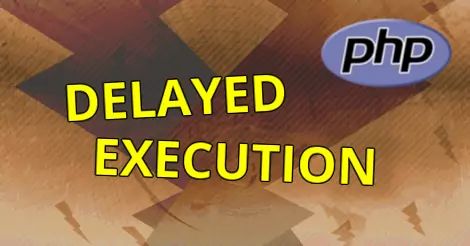
It is very common to want to add a delay in execution of a script. In PHP, this can be done using the different sleep functions.
The native sleep() function will pause execution of the script for the specified amount of time in seconds. It is also possible to pause execution in nanoseconds using time_nanosleep(), or microseconds with usleep().
There is no native function for Milliseconds, but you can create your own function.
To sleep for ten seconds, you would simply type the following in your script:
echo "This is the beginning\n";
sleep(10);
echo "And this is the end\n";
Task scheduling with PHP sleep
It is generally a bad idea to schedule execution from PHP scripts. Cron does a much better job at scheduling.
That is not to say that it can not be done. You just need to be very careful, making sure that your script is actually running, and being careful not to run multiple copies. You do not want your scripts to miss execution because your scheduler is either blocked or crashed, and you certainly to not want to run tasks more often than intended.
You would probably also want to avoid using HTTP requests to call the scheduled scripts, in case of network failure. But, it depends on the requirements of the process.
To avoid accidentally running multiple instances of your scheduler, you may want to use a simple flock to block or reject attempts to run the scheduler while it is already running.
Max execution time
Sleep functions do not count towards a scripts max_execution_time. A way to test this would be to run the below script from a browser:
$starttime = time();
time_sleep_until(time()+60);
// Do Stuff Here
$finishtime = time();
header('Content-Type: text/plain; charset=UTF-8');
echo $finishtime-$starttime . " seconds\n\n";
echo 'max_execution_time: '. ini_get('max_execution_time') . "\n";
The output should be ~59 seconds.
In addition, scripts running from the command line does not have a max execution time.
Time table
The following table shows how many seconds there is over different time spans:
1 day | 86400 |
1 weak | 604800 |
1 month | 2629746 |
1 year | 31556952 |
Tell us what you think: