PHP: Calculating Exponential Growth
Why the caret character is not working in exponential growth calculations in PHP, and what to use instead.
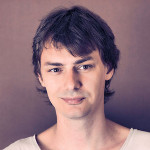
By. Jacob
Edited: 2020-07-27 10:47
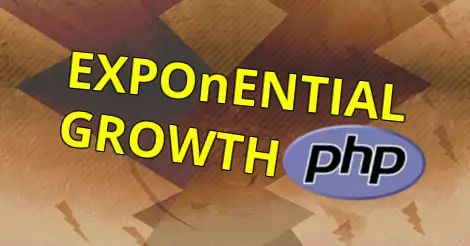
When calculating exponential growth in PHP, the syntax is a bit different than in standard math; basically, instead of using the caret ^ character to define the exponent, the double asterisk operator ** is used.
The double asterisk is an arithmetic operator used for working with exponentiation.
In order to calculate exponential growth in PHP, we should write the formula like this:
echo 1000*1.1**1; // 1100
Or, using variables:
$s = 1000; // Starting number
$r = 0.10; // The growth rate (10%)
$n = 1; // The exponent
$end_number = $s*(1+$r)**$n;
echo $end_number; // 1100
Addition, Subtraction, and Multiplication still function the same way as when doing math in a spreadsheet or a calculator. I.e.:
$a =10;
$b = 10;
echo $a + $b; // 20
echo $a / $b; // 1
echo $a * $b; // 100
These are all part of the arithmetic operators in PHP. If you are a non English speaker, then this may be one of those cases where it is hard to find an answer when searching in Google.
I did not personally think of searching for "arithmetic", instead I searched for "math operators"; it took me a while to figure out why the caret character was not working as I expected.
Tell us what you think: