PHP: Check if Number is a Decimal Number
This tutorial shows how to check if a number is a decimal number using the PHP floor function.
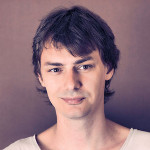
By. Jacob
Edited: 2020-11-02 15:57
There is no build-in function in PHP to check if a number is a decimal, but we can make our own using the floor function.
The following code shows whether a provided number is a decimal number:
function is_decimal($n) {
// Note that floor returns a float
return is_numeric($n) && floor($n) != $n;
}
To use this with an if statement:
if (is_decimal(20.5)) {
echo 'We are dealing with a decimal number.';
} else {
echo 'The provided number is not a decimal number.';
}
Check if a number is a decimal
The function is very compact, and perhaps a bit hard to read, so let us take a moment and go through the different parts.
First we check if the provided input is a number, we do this using the is_numeric function; this function either returns true or false depending on the result.
We will also need to check if the number is a decimal, so we use a logical operator (&&) for "and"; the function will therefor only return true if the expressions on both sides of the && operator returns true, otherwise it will return false.
Below is a few examples to show how the function can be used:
var_dump(is_decimal(200)); // bool(false)
var_dump(is_decimal(200.0)); // bool(false)
var_dump(is_decimal(200.2)); // bool(true)
Tell us what you think:
Thanks! checking for is_numeric and floor is simple and effective