PHP: How to Find Common Multiples
Lean to find the common multiples between two numbers in PHP; the tutorial starts by teaching you about multiples, then moves on to common multiples.
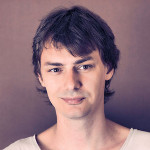
By. Jacob
Edited: 2020-11-08 12:23
In basic math, a multiple is the result of multiplying a number with an integer; for example, the numbers 4, 6, 8 are all multiples of 2 — we arrive at each number by multiplying 2 with an integer (n).
In this tutorial I will show you how to create a few PHP functions that returns multiples and common multiples as an array.
In basic math, to get the multiples of a number, we can just keep multiplying the number repeatedly with an integer:
2*2=4
2*3=6
2*4=8
The integer should be incremented after each multiplication; note that this can continue on infinitely — or until your heart stops beating.
So how do we do this in PHP? Well, the most straight forward way would be to simply carry out a series of multiplication calculations, as you would have done it on a piece of paper.
In PHP we do not need to write out all the multiplications; instead we can use a looping mechanism to repeat a piece of code, and automatically increment the integer used in the multiplication. Each multiple can then be stored in an array for later use.
The below function can be used for this purpose:
function get_multiples(int $a, int $n=10) {
$multiples_arr = array();
for ($i=1; $i <= $n; $i++) {
$multiples_arr[] = $a * $i;
}
return $multiples_arr;
}
By default, this will return the 10 first multiples of a supplied number, and the $n variable can be adjusted if more are needed.
For example:
$multiples_of_two = get_multiples(2, 11); // Get 11 first multiples of 2
var_dump($multiples_of_two);
Output:
array(11) {
[0]=>
int(2)
[1]=>
int(4)
[2]=>
int(6)
[3]=>
int(8)
[4]=>
int(10)
[5]=>
int(12)
[6]=>
int(14)
[7]=>
int(16)
[8]=>
int(18)
[9]=>
int(20)
[10]=>
int(22)
}
Common Multiples
A Common Multiple is a multiple that is shared by two or more numbers. Finding all the common multiples of two numbers can be done by maintaining a list of all the multiples for each number, and then compare the lists to find the numbers that occur in both; this means we need to store the numbers as two separate arrays.
We can even re-use the function I introduced earlier, and do like this:
$multiples_of_two = get_multiples(2);
$multiples_of_four = get_multiples(4);
Now we got a list of multiples stored in the $multiples_of_two and $multiples_of_four variables; all we have to do now is to compare them.
To compare the arrays, we can use a foreach loop. But, we should really wrap this whole thing in a function so we can call the code whenever we need:
function find_common_multiples(int $a, int $b, $n=10) {
$a_multiples_arr = get_multiples($a, $n);
$b_multiples_arr = get_multiples($b, $n);
$common_multiples = array();
foreach ($a_multiples_arr as $a_multiple) {
if (in_array($a_multiple, $b_multiples_arr)) {
$common_multiples[] = $a_multiple;
}
}
return (!empty($common_multiples)) ? $common_multiples : false;
}
Now, to get a list of the common multiples between two numbers, we can just call this function:
$common_multiples = find_common_multiples(2, 4);
Least- and highest common multiple
Since we have stored the common multiples in an array, we can also easily return both the Least Common Multiple (LCM), and
PHP comes with build-in functions to return the last and first elements from an array, which will correspond to the LCM and HCM of the two numbers:
$common_multiples = find_common_multiples(2, 4);
$lcm = current($common_multiples);
$hcm = end($common_multiples);
echo 'Least common multiple: ' . $lcm . "\n";
echo 'Highest common multiple: ' . $hcm. "\n";
Output:
Least common multiple: 4
Highest common multiple: 20
Lists of multiples
Here is a few pre-calculated lists to help you verify your results.
Multiples of two:
Number | Integer |
---|---|
2 | 1 |
4 | 2 |
6 | 3 |
8 | 4 |
10 | 5 |
12 | 6 |
14 | 7 |
16 | 8 |
18 | 9 |
20 | 10 |
Multiples of four:
Number | Integer |
---|---|
4 | 1 |
8 | 2 |
12 | 3 |
16 | 4 |
20 | 5 |
24 | 6 |
28 | 7 |
32 | 8 |
36 | 9 |
40 | 10 |
Multiples in common:
Common Multiples between 2 and 4 |
---|
4 |
8 |
12 |
16 |
20 |
Tell us what you think: