Create, and Delete Files and Directories
How to do disk operations, such as creating and deleting files, in PHP.
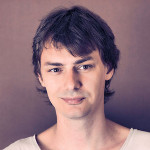
By. Jacob
Edited: 2019-09-30 01:58
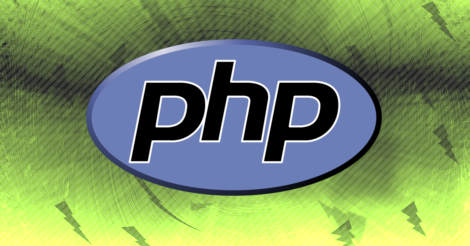
When Creating files and folders in PHP you should first make sure that your web server has the required permissions. In Linux, for the directory you are going to write to, the permissions should be set to 774.
In PHP, writing new files is done using a combination of the fopen, fwrite, and fclose functions. But, you can also use file_put_contents, which is just a wrapper for fopen, fwrite amd fclose. In this tutorial, we will be focusing on the first mentioned functions.
Examples:
mkdir("/path/to/my/dir", 0774); // Create Directory
rmdir('dir'); // Remove Directory
unlink('MyFile.html'); Delete file
rename("/folder/old-file-name.ext", "/folder/new-file-name.ext"); // Rename file or directory
// Write to file. If it does not exist attempt to create it.
$file = fopen($pathtofile, "w");
if((fwrite($file, $content) == false) && (!file_exists($pathtofile))) {
fclose($file);
echo 'File not written, check permissions!';
} else {
fclose($file);
echo 'Success!';
}
Create, write, and delete files
The function names are a little misleading, as they indicate that a file needs to exist before you can write to it. This is not the case. But, it actually depends on the mode parameter of fopen. In this script we are using "w", therefor, If a file does not exist, it will automatically be created.
A small script to create a file can look like the below:
$pathtofile = $_SERVER["DOCUMENT_ROOT"] . 'files/'; // Should result in something like: /var/www/MySiteName/files/
$content = 'Blah blah';
$file = fopen($pathtofile, "w");
if((fwrite($file, $content) == false) && (!file_exists($pathtofile))) {
fclose($file);
echo 'File not written, check permissions!';
} else {
fclose($file);
echo 'Success!';
}
The if check in the above script checks if the file-create was successful. Due to problems with fwrite return values, we also have to check if the file exist after creating it. This is done with the file_exists function. We need both checks, because if the file already exists when trying to create it, and it for some reason fails, we would likely want to know about it.
The problem with fwrite is that it returns "0" if the $content is empty, which also validates to false when checked with if(fwrite(...)), and since we might want the ability to create empty files, we will need to account for this with file_exists.
Note. There can be other reasons why fwrite fails, but it is most likely because the user running the server does not have the necessary permissions.
The mode parameter – the "w" parameter of the fopen function – is used to control which mode to open the file in. In this case the file is opened for writing. If the file does not exist, it will be created. Finally, if it already exists, it will be overwritten. You can read more on different modes in the PHP reference on fopen.
Finally, the path accepted by fopen for local files is the server-sided full path, and not a relative path. You can use $_SERVER["DOCUMENT_ROOT"] to get the full path of the directory where the script is located.
Deleting a file is done with unlink:
unlink('MyFile.html');
Renaming files or directories
Renaming files or directories is done with the rename function. For example, to rename a file in the current working directory – without moving it to another directory – you should do like demonstrated in the below example:
rename("/folder/old-file-name.ext", "/folder/new-file-name.ext");
Keep in mind that the rename function operates from the Working Directory. The above example is using root relative paths to access, rename and/or move files.
Creating and deleting directories
Directories can be made with the PHP mkdir function, and deleted with rmdir. The below code creates a directory with the 774 permissions:
mkdir("/path/to/my/dir", 0774); // Creates the directory
By default, mkdir will create the directory with 777 permissions, which gives full access to all users on your server (read, write, and execute). Note the leading zero in the second parameter, (this is not needed for chmod).
You likely want something like 774, to prevent the directory from being too open.
Also, the $_SERVER["DOCUMENT_ROOT"] variable can be used when determining the full path.
To remove the directory, simply use rmdir:
rmdir('dir'); // Removes a directory
Permissions
Do not try to change permissions from your file manager (I.e. Nautilus/Files), as this often seem to be broken. Hopefully it will be fixed in the future, so be careful. Instead open a terminal and enter below commands:
chown -R USER:GROUP [DIRECTORY]
chmod 774 -R [DIRECTORY]
USER will become the owner of the directory, and other users, who are members of GROUP will also have full access. (I.e User:Group:Other, each corresponds with a number = xxx)
If you want both read and write access, 4 is added with 2. If you also want permission to execute files, we get 7. Hence, 774. The last number (4) controls permissions for users not in the group.
read | (view contents, i.e. ls command) | r or 4 |
write | (create or remove files from dir) | w or 2 |
execute | (cd into directory) | x or 1 |
Tell us what you think: