PHP: Check If A File Was Included
How to get a list of included files and know if a certain file was included by PHP.
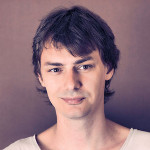
By. Jacob
Edited: 2020-11-14 10:58
Sometimes you may want to know whether a file was included in a PHP script or CMS, to do that we can use the get_included_files function; this function will return an array of absolute paths to the files that has been included by PHP.
The returned list is not always a complete list, since it is possible to include files at different points in the execution process. If a file is included by a function or method, and this has not been called yet, then the file will also not exist in the included files list.
When working with Wordpress, you may want to use a hook, as that might help get a more complete list of files; you should read more about this in our Wordpress tutorials.
We can display the output of the get_included_files function like this:
print_r(get_included_files());
Or, if you want to show the output as plain text:
header('content-type: text/plain; charset=UTF-8');
print_r(get_included_files());exit();
Checking if a file was included
The get_included_files function will produce a list of included files, regardless of whether they have been included using require, include, require_once, or include_once
To understand the difference between these different statements you can read this article: Include, Require, Include_once, and Require_once
There are two easy approaches when you want to check if a file has been included, one is to use the get_included_files function, which is the recommended approach, and another is to use the include_once statement — but the latter does not work well, since it triggers a fetal error if a file has already been included.
Since get_included_files returns an array of paths, we can just loop over the array in order to check if the string contains the file path we are looking for:
$list_of_fules_arr = get_included_files();
$string_to_look_for = 'shortcodes.php';
foreach ($list_of_fules_arr as $file_path) {
if (false !== strpos($file_path, $string_to_look_for)) {
echo 'Found: ' . $file_path;exit();
}
}
To instead show all included files:
foreach ($list_of_fules_arr as $file_path) {
echo $file_path . "\n";
}
Extract the file name from the file path
You will often only need to know the file name of the included files, but since a system might indeed include multiple files with the same name — at different locations — this is not recommended.
The base path should be safe to remove though, and this way you can still tell the difference between two files that happen to have the same name. To do that, we first need to determine the base path, which can only be done from the composition root (I.e. index.php) relying on the document root is not consistent. Instead we should do it manually, like this:
define('BASE_PATH', rtrim(preg_replace('#[/\\\\]{1}#', '/', realpath(dirname(__FILE__))), '/') . '/');
Now we have defined a constant called BASE_PATH that we can use to "cut off" the irrelevant /var/www/some_site/... part of the included file paths:
foreach ($list_of_fules_arr as &$file_path) {
$file_path = str_replace($file_path, '', BASE_PATH);
}
print_r($list_of_fules_arr);
Output:
Array
(
[0] => /index.php
[1] => /wp-blog-header.php
[2] => /wp-load.php
[3] => /wp-config.php
[4] => /wp-settings.php
[5] => /wp-includes/version.php
[6] => /wp-includes/load.php
...
)
Tell us what you think: