PHP: Include, Require, Include_once, and Require_once
Tutorial on how to use PHPs include, include_once and require.

Edited: 2020-10-27 10:49
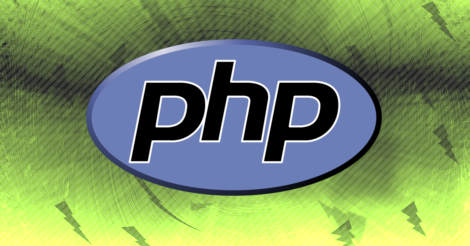
PHP Includes are useful when writing, simple, reusable, and maintainable code.
As a general rule, you should aim to repeat yourself as little as possible; this rule is also known as the Don't Repeat Yoursel (DRY) rule, and for this purpose, includes is just one tool to help you better organize code and avoid code repetition.
There are a number of different ways to include files in your scripts. In PHP, we have the following: Include, include_once, require, and require_once. Each of these have their own use case, which will be explained in this tutorial.
When in doubt, you should always use either require_once or require, as these will help you more easily debug a broken path, and the _once version will help you to avoid having files included multiple times and causing errors — something that might happen if the CMS or framework is calling your code multiple times.
When working with includes, the best way to determine the base path is probably by using the following somewhere in your composition root (I.e. Your index.php file):
define('BASE_PATH', rtrim(preg_replace('#[/\]{1}#', '/', realpath(dirname(__FILE__))), '/') . '/');
See also: Determining the Document Root or Base Path in PHP
This will create a constant named BASE_PATH that we can use throughout the code. Of course, it is not recommended to rely too much on constants in an Object Orientated context; instead you might want to consider passing the base path through Dependency Injection.
Include and Include_once
Include will attempt to include whatever file specified, regardless if it exists or not. If the file was not found, the script will continue running anyway.
<?php
include "whatever.php"; // Includes whatever.php
?>
The include_once statement will only include the file, if it has not already been included before in the application.
<?php
include_once "whatever.php"; // Includes whatever.php
include_once "whatever.php"; // Won't do anything, since the file was already included.
?>
If a file was not found, a warning is created. You will only be able to see this warning if you have enabled error reporting for your script.
Warning: include(settings.php): failed to open stream: No such file or directory in path on line 50 Warning: include(): Failed opening 'settings.php' for inclusion (include_path='.;path') in path on line 50
Require, and require_once
Require indicates that the file is required, and that the script should stop executing if the file was not found.
<?php
require "whatever.php"; // Includes whatever.php
// The rest of the script, will only be finished if the above require succeeds
?>
A warning will be issued, depending on your error settings. If the warning does not show up in the browser, you can usually find it in the server log files.
Again, Require_once makes sure that the file will only be included once in your application.
Determining the inclusion path
A problem that many programmers run into, is that base path can be difficult to work out.
In Linux systems, the base path is usually absolute. A way to easily determine the path, without having to write the entire path for your web-servers directory, is to use $_SERVER["DOCUMENT_ROOT"], but this might not work in all cases, since the content can be inconsistent.
Ideally a directory should always end with a slash (/), as this will indicate to developers that we are working with a directory path, and the developer can trust that the slash is always present. With DOCUMENT_ROOT, the slash might not be present, and that can cause issues.
A better way is therefor to define a base path variable yourself:
define('BASE_PATH', rtrim(preg_replace('#[/\]{1}#', '/', realpath(dirname(__FILE__))), '/') . '/');
This can then be used when including files throughout your project:
require BASE_PATH .'lib/db_client_class.php';
The above would correspond to the following path in Ubuntu, running the Apache HTTP Server:
/var/www/website_name/lib/db_client_class.php
And this needs to be done once, typically in the composition root (often that would be the index.php file). See also: Determining the Document Root or Base Path in PHP
Tell us what you think: