Rename All Files in a Directory
I needed a script to quickly rename all files in a directory, so I came up with this elegant PHP solution.
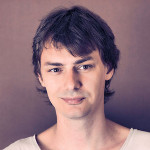
By. Jacob
Edited: 2019-09-30 01:52
I created this short php script to quickly rename all the files in a directory.
To control which part'(s) of the file to rename, you simply need to modify the regular expression used with preg_match.
The script can be run from a terminal window using php. I.e.: php rename.php
When used in a terminal, the script will output a list of files it has renamed. If you want to run the script from a browser, you should first send the appropriate mime type for plain text. I.e:
header("Content-Type: text/plain; charset=utf-8");
The script will not check for write permissions. You can do this yourself using is_writable()
The script will only rename files. To also rename directories, you should uncomment the is_dir() if check.
The PHP script itself:
$all_object = '';
$objects = array_diff(scandir('.'), array('..', '.')); // Removes "." and ".." from the array, since they are not needed.
foreach($objects as $object) { // Loops through the $objects array
if (is_dir($object) == false) { // Check if $object is a directory (to only rename files)
if (preg_match('/(.+)\-[a-z0-9_-]+(\.mp3)/i', $object, $matches)) {
// The files to-be-renamed will match the pattern you provide for preg_match
$all_object .= $matches[1] . $matches[2] . "\n"; // Add each $object to string for later output
if(rename($object, $matches[1] . $matches[2]) == false) {
// Attempt to rename the current $object, exit on failure.
echo "\nFailed to rename file'(s)\n";exit();
}
}
}
}
echo "\n"; // New line
echo 'Renamed: ' . $all_object;
echo "\n";
Tell us what you think: