Installing and Using GD Library with PHP
How to install the GD library and use it to write text to an image.
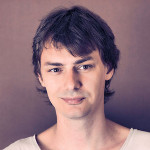
By. Jacob
Edited: 2020-05-30 10:26
The GD library makes it possible to work with images in PHP, but it might need to be installed separately in order to work. If you are using a Ubuntu or Debian based Linux distribution, this is fairly straight forward using apt install, but you need to make sure to install the package that corresponds with your PHP version.
To find out your PHP version, type the following in your terminal:
php -v
In general, you will often be able to guess the package name, since there is a system to it; at least there is with PHP related packages. For example, to install GD library, you would try something like:
sudo apt install php7.4-gd
If you are using PHP 7, you may instead need to type:
sudo apt install php70-gd
Note. You may also search for the right package. This is done with apt-cache search php7
Once the GD package is installed you should also restart your server:
sudo service apache2 restart
Writing some text to an image
To see if the GD library was properly installed, we can try to create an image containing a simple text string.
To create the image, we use the imagecreate function, while adding the text itself is done with the imagestring function.
The imagecolorallocate is used to add the colors used in our image. Note that the first call to this function sets the background color.
// Create a 300*100 image
$img = imagecreate(300, 100);
// Backround and Text color
imagecolorallocate($img, 243, 243, 243);
$textcolor = imagecolorallocate($img, 33, 33, 33);
// Writa the text at given coordinates
imagestring($img, 5, 120, 40, 'Beamtic', $textcolor);
// Output the image
header('Content-type: image/png');
imagepng($img);
imagedestroy($img);
exit();
If CAPTCHAS is your thing, then you now have a solid starting point to create a basic CAPTCHA system that does not rely on third party services.
Tell us what you think: