The HTML Mime Type
The HTML Mime Type is the default used by PHP, and you need to actively change it to deliver other types of content.
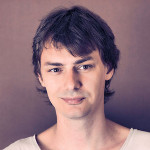
By. Jacob
Edited: 2020-07-04 12:33
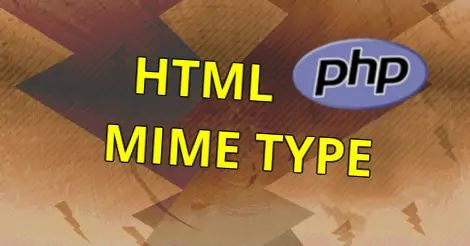
The standard Content Type of HTML files is text/html, and the default file extension is .html.
Web pages on the internet will typically be HTML pages, but often times the file extension will not be used, since the content type is not of much relevance to users. It is also possible to not use file extensions with image files, but this is bad, since it might confuse most users.
When using PHP, the HTML content-type is used by default. This means, if you echo 'Just some irrelevant text';, or escape in and out of PHP using the start and opening tags, text/html will be used by default.
A HTTP response for a HTML page looks like this:
HTTP/1.1 200 OK
content-type: text/html
content-length: 832
Even though the default content type in PHP is HTML, there may still be times when we want to manually send the headers; to do so, we may use the header function like this:
header('content-type: text/html');
echo $html_content;
exit();
Var_dump and HTML
There may be times when we want to output the content of variables as HTML, but PHP's build-in var_dump function will not preserve whitespace by default.
Luckily, we can create our own function for outputting both text/html and text/plain.
I usually have this function placed in my global scope somewhere:
function dumpHTML($input) {
header('Content-Type: text/html');
echo '<pre>';
var_dump($input);
echo '</pre>';
exit();
}
This function will take the content of a variable, and dump it in a pre element, which will preserve whitespace and line breaks.
I have a similar function for when I just want text/plain returned:
function dumpText($input) {
header('Content-Type: text/plain');
var_dump($input);
exit();
}
Tell us what you think: