PHP: http_response_code vs header
Article documenting the reasons to use Http_response_code instead of sending raw HTTP response codes in PHP.
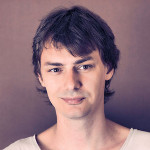
By. Jacob
Edited: 2020-08-29 10:34
The main benefit of using the http_response_code function, is that we can send HTTP response codes, without having to think about the protocol version used by the client. If instead we send raw response codes with the PHP header function, we will need to manually determine the protocol version.
Many PHP applications will incorrectly send a raw HTTP header response code, without properly determining the protocol the client is using. This can in rare cases lead to problems.
Hard-coding the protocol, such as HTTP/2.0 or HTTP/1.1 is unnecessary, and increases the chance of errors—instead we should allow PHP to determine the protocol automatically by using the http_response_code function.
Another benefit is that you minimize risk of misstyping the response header field when manually sending headers from PHP.
To send a HTTP response code with PHP, we could do like shown in the below example:
http_response_code(200);
echo '<p>Hallo World!</p>';
Sending raw HTTP headers with PHP
If you still want to send raw headers, be sure to use a function like the below to determine the HTTP protocol version used by the client:
function set_protocol() {
$supported_protocols = array(
'HTTP/2.0' => true,
'HTTP/1.1' => true,
'HTTP/1.0' => true,
);
$protocol = $_SERVER["SERVER_PROTOCOL"];
if (!isset($supported_protocols["$protocol"])) {
$protocol = 'HTTP/1.0';
}
return $protocol;
}
Tell us what you think: