PHP: headers_list
The headers_list() function will return a list of headers sent by PHP, or headers that are ready to be sent by PHP.
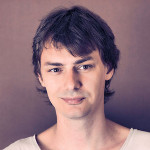
By. Jacob
Edited: 2020-08-29 10:46
The headers_list function will returns an array of server response headers; it should not be confused with the reserved variable, $http_response_header — the reserved variable contains the response headers from a HTTP request, while headers_list contains the local response headers set by PHP.
The headers_list function will return raw headers as an indexed array; if instead an associative array with key and value pairs is desired, we can also parse the response headers.
This function will only return the headers that are sent, or ready to be sent, from PHP — not the response headers that are controlled by the web server.
This function will not work from command line scripts.
If calling the headers_list function like this:
header('test: testing');
var_dump(headers_list());
We will get:
array(1) {
[0]=>
string(13) "test: testing"
}
How to parse the headers_list() array
It will often be more useful to create an associative array of key and value pairs when juggling with HTTP headers. To do this, we should parse each header and pick out the key and value using PHP's string functions.
As established in the tutorial on parsing HTTP headers, we can do this very efficiently using the build-in explode function:
$headers = array();
$response_headers = headers_list();
foreach ($response_headers as $value) {
if(false !== ($matches = explode(':', $value, 2))) {
$headers["{$matches[0]}"] = trim($matches[1]);
}
}
print_r($headers);
Output:
Array
(
[test] => testing
)
Note. We did not attempt to extract the HTTP status code since it is not contained in the array returned to us by headers_list
Links
- headers_list - php.net
Tell us what you think: