The REQUEST_METHOD superglobal in PHP
It is sometimes useful to know the HTTP request method, and PHP makes this easy via the REQUEST_METHOD super global..
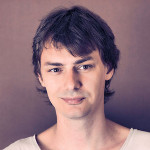
By. Jacob
Edited: 2021-05-21 07:36
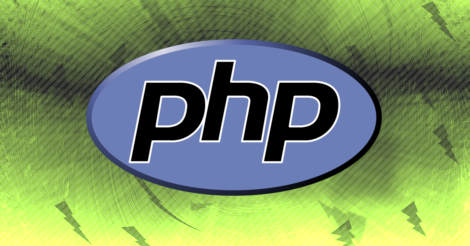
To check the request method you may use the $_SERVER['REQUEST_METHOD'] variable, the $_SERVER is a PHP superglobal that is available to you at any time, even inside functions and classes.
To use the REQUEST_METHOD variable you could just echo its contents, but it is probably more useful in a switch or if statement.
Request types:
- GET
- POST
- HEAD
- PUT
- DELETE
- PATCH
Example:
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
echo 'The request was POST';
exit();
} else {
http_response_code(405);
header('Allow: GET, HEAD');
echo '<h1>405 Method Not Allowed</h1>';
exit();
}
When to use REQUEST_METHOD
The REQUEST_METHOD variable may be used whenever you need to determine the HTTP request type.
For example, if you know your application only accepts user input via HTTP post requests, it is recommended to block other types of requests, and inform the user that the request is not valid.
It can be used as part of the server-side validation of user input, before attempting to validate the input itself.
The REQUEST_METHOD variable is filled out by PHP based on the HTTP request type, and can therefor be safely used without validation. There should be no risk of injection attacks in this variable.
We can not simply check if $_POST and $_GET is empty, since they are always defined, even if all the HTML form fields are empty.
Tell us what you think: