Showing a Random Image in PHP
This tutorial explains how to create a PHP script to show a random image in a browser.
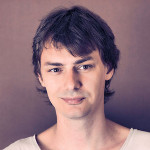
By. Jacob
Edited: 2021-02-13 14:18
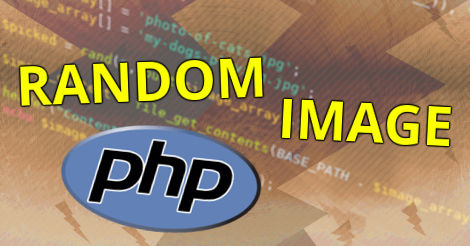
In this tutorial, we will show how to show a random image with PHP. The procedure is actually quite simple. You have an array with a list of images, and then you use the build-in rand() function to randomly pick a image in the array based on the array-key.
Images are loaded from the file system using file_get_contents() (or the other file- functions). The first is probably easier to use, so we will stick with that in this tutorial. Once we have used file_get_contents() to load the image, we can send it to the browser with the appropriate HTTP response headers, and since we are working with .jpg images, we should use the image/jpeg mime-type. The complete script is shown below:
define('BASE_PATH', rtrim(preg_replace('#[/\\\\]{1}#', '/', realpath(dirname(__FILE__))), '/') . '/');
$image_array = array();
$image_array[] = 'photo-of-cats.jpg'; // Key starts at "0"
$image_array[] = 'my-dogs-playing.jpg'; // Key will automatically be assigned "1"
$picked = rand(0,count($image_array)-1); // maximum value is derived by counting $image_array
$image_content = file_get_contents(BASE_PATH . $image_array["$picked"]);
header("Content-type: image/jpeg");
echo $image_content;
How to show a random image with PHP
1. First we need to create the array containing the images. This can either be done manually, or automatically, and both is actually quite simple. But, for simplicity, we will be sticking with manual approach in this tutorial.
$image_array = array();
$image_array[] = 'photo-of-cats.jpg';
$image_array[] = 'my-dogs-playing.jpg';
2. Next, the rand() works by generating a random number between two values which we can provide as parameters. The second parameter is the maximum, and is obtained by counting the number of images found in $image_array. But, since the array starts at "0", count() will return an incorrect value – we can correct the count by subtracting "1". I.e:
$picked = rand(0,count($image_array)-1);
3. Now, to pick a random image from the $image_array array, we may simply use the $picked number we generated with the rand() function from before.
$image_content = file_get_contents(BASE_PATH . $image_array["$picked"]);
Note. We are using the BASE_PATH to create the exact path to the image on the server. Depending on your own server configuration, you may need to adjust this.
4. The final step is to deliver the correct mime type of the image via the HTTP response headers, we will do this using the PHP header() function:
header("Content-type: image/jpeg");
5. Finally, we may echo the image content, after having delivered the right mime type in the headers:
header("Content-type: image/jpeg");
echo $image_content;
Note. Never deliver headers after delivering content to the browser, as it will cause an error I.e.:
header("Content-type: image/jpeg");
echo $image_content;
Tell us what you think: