Creating a Fading Slideshow with JavaScript
How to create a slideshow that uses a fade transition with JavaScript.
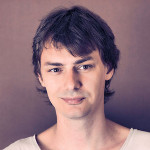
By. Jacob
Edited: 2019-09-11 16:35
Based on this: JavaScript fade effect script
In this tutorial, we will be be creating a slideshow script that will continuously fade between a series of images or HTML elements.
Each image is included in its own div element which is then faded. All content within the div should be affected by the effect. The divs themselves will be hidden trough the use of the CSS display property.
The StartFade Function
The StartFade function is optional if you are using the unload event – but if it's possible for users to trigger the effect more than once, then it might be a good idea to include the starter function, to avoid that the effect overlaps.
First declare the variable which is used to check if the function is already running:
var running = 0 /* Used to check if fade is running */
And here is the start function for our fade effect.
function StartFade() {
if (running != 1) {
running = 1
fadeOut()
}
}
This solves a problem were clicking the button multiple times breaks the effect. Next we will work on the slideshow.
JavaScript Slideshow Fade
First we should hide the slice elements with CSS, this will be done by using the display property. The below will be added to our existing CSS code:
#Slideshow div {display:none;}
#Slideshow #slice1 {display:block;}
And now for the element counters. They should be declared before we use them. We will use the counters to determine which elements to show and hide.
var iEcount = 1 /* Element Counter */
var iTotalE = element.getElementsByTagName('div').length;
function FadeIn() {
for (i = 0; i <= 1; i += 0.01) {
setTimeout("SetOpa(" + i +")", i * duration);
}
if (iEcount == iTotalE) {
iEcount = 1
document.getElementById("slice" + iEcount).style.display = "block";
document.getElementById("slice" + iTotalE).style.display = "none";
} else {
document.getElementById("slice" + (iEcount + 1)).style.display = "block";
document.getElementById("slice" + iEcount).style.display = "none";
iEcount = iEcount+1
}
setTimeout("fadeOut()", (duration + showtime));
}
You can easily output whatever is to run in the slideshow from the serverside, but that will not be covered in this Article.
<div id="Slideshow">
<div id="slice1">Slice1
</div>
<div id="slice2">Slice2
</div>
<div id="slice3">Slice3
</div>
</div>
Whatever is to be run in the slideshow, should be included in the slice elements. It can almost be anything, including images and text – and by the way, you can easily add more slice elements without having to modify the script.
It may be a good idea to stay around 3-5 slices – I.e. 3-5 latest phothos/news or whatever.
The Full Slideshow Script Example
Do not bother trying to put the above together, it most likely wont work. You can use the full script from here, and even try it directly in your browser.
<!DOCTYPE html>
<html lang="en-US">
<head>
<title>JavaScript Fade - beamtic Example</title>
<style type="text/css">
#Slideshow {
opacity: 1.0; /* CSS3 */
-moz-opacity: 1.0; /* Older versions of Firefox */
-khtml-opacity: 1.0; /* Older versions of Safari */
filter: alpha(opacity=100); /* Internet Explorer */
}
#Slideshow div {display:none;}
#Slideshow #slice1 {display:block;}
</style>
</head>
<body>
<div id="Basement" style="width:90%;margin:auto;">
<button onClick="StartFade()">Click Me</button>
<div id="Slideshow">
<div id="slice1">Slice1
</div>
<div id="slice2">Slice2
</div>
<div id="slice3">Slice3
</div>
</div>
</div>
<script type="text/javascript">
var element = document.getElementById("Slideshow");
var duration = 3000; /* fade duration in millisecond */
var hidtime = 2000; /* time to stay hidden */
var showtime = 2000; /* time to stay visible */
var running = 0 /* Used to check if fade is running */
var iEcount = 1 /* Element Counter */
var iTotalE = element.getElementsByTagName('div').length;
function SetOpa(Opa) {
element.style.opacity = Opa;
element.style.MozOpacity = Opa;
element.style.KhtmlOpacity = Opa;
element.style.filter = 'alpha(opacity=' + (Opa * 100) + ');';
}
function StartFade() {
if (running != 1) {
running = 1
fadeOut()
}
}
function fadeOut() {
for (i = 0; i <= 1; i += 0.01) {
setTimeout("SetOpa(" + (1 - i) +")", i * duration);
}
setTimeout("FadeIn()", (duration + hidtime));
}
function FadeIn() {
for (i = 0; i <= 1; i += 0.01) {
setTimeout("SetOpa(" + i +")", i * duration);
}
if (iEcount == iTotalE) {
iEcount = 1
document.getElementById("slice" + iEcount).style.display = "block";
document.getElementById("slice" + iTotalE).style.display = "none";
} else {
document.getElementById("slice" + (iEcount + 1)).style.display = "block";
document.getElementById("slice" + iEcount).style.display = "none";
iEcount = iEcount+1
}
setTimeout("fadeOut()", (duration + showtime));
}
</script>
</body>
</html>
Tell us what you think: