If statements using colon and semi-colon style in PHP
How to use the alternative syntax of writing if statements in PHP to perform conditional execution of code.
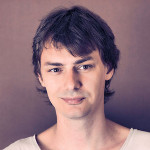
By. Jacob
Edited: 2021-02-14 03:09
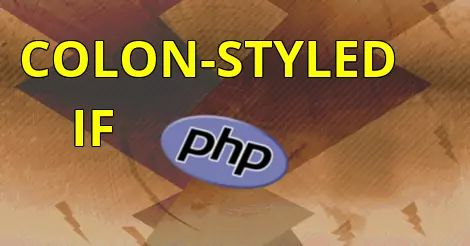
In PHP, there is an alternative syntax for writing if statements when performing conditional execution of code, allowing us to use colon and semi-colon when writing the if statement.
This syntax is using endif keyword to finish the if statement.
A single if block will look like this:
if ('John' == $name):
echo 'Hallo Rasmus :-)';
endif;
Including an else case in the statement:
if ('Zeev' == $name):
echo 'Hallo Zeev :-)';
else:
echo 'Sorry. I do not know you.';
endif;
Finally, we may also use the elseif keyword:
if ('Andi' == $name):
echo 'Hallo Andi :-)';
elseif ('Rasmus' == $name):
echo 'Hallo Rasmus!';
elseif ('Zeev' == $name):
echo 'Hallo Zeev!';
endif;
Short-hand style
Of course, colons are also used as an else substitute in one-line if statements.
This is how to check if a variable is empty in a nice little one-liner:
// Assign a $_POST variable if it was not empty
$name = if (!empty($_POST['name'])) ? $_POST['name'] : null;
This is very readable and useful when you have a lot of user-input that you need to validate.
Tell us what you think: