If and else conditional statements in PHP
How to create a simple If- Else Statement in PHP to perform conditional execution of code.
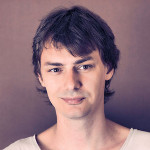
By. Jacob
Edited: 2021-02-14 04:24
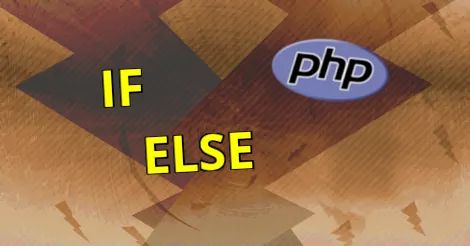
This tutorial discusses how to write a simple if, else statement in PHP.
We can either choose to use the "more readable" form, complete with parentheses and curly brackets, or we can use a shorter version. In this tutorial I will show you how to do both. Which one is the better one to use will depend mostly on the circumstances.
If statements is a concept that is shared across programming languages. So, if you know how to use them in one language, it will generally be much easier for you to use them in another.
A single if statement looks like this:
if ($some_variable == $another_variable) {
echo 'The variables matched!';
} else {
echo 'The variables did not match';
}
Or, using Colon and semi-colon
if (100 == $age):
echo 'You have reached one hundred years of age.';
else:
echo 'Still not there yet...';
endif;
Evaluation order
Conditional statements are evaluated left to right, this means that we can both check that a variable is set, as well as validate it in the same statement. The condition closest to the left will be evaluated first:
if (empty($_POST['email']) || !filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo 'The email field was either empty or invalid. Exiting.';
exit();
}
// In case the email field was not empty and valid, we continue
echo 'Thank you for subscribing to our newsletter.';
If you have multiple of these conditionals, you might want to wrap them in an extra pair of parentheses:
if (
(!empty($_POST['email'])) // If not empty, perform the next check
|| (!filter_var($email, FILTER_VALIDATE_EMAIL)) // If valid, do next check
|| (!empty($_POST['comment'])) // This is the final check
) {
echo 'Something was not filled out correctly. Exiting.';
exit();
}
Guard clauses
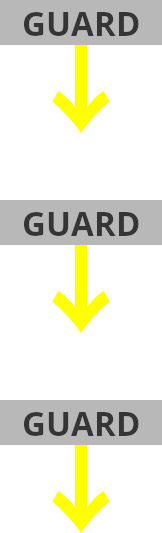
The else clause is rarely needed, and often just lowers the readability of the code. Instead of relying on else and else if in the code, a popular alternate approach is to create so-called guard clauses.
In programming, a guard clause is a structure that either stops the execution or allows it to continue. It can be in the form of an if statement, but it is also possible to use functions for this purpose. The idea is to limit the amount of nesting as much as possible, and instead replace nested conditionals, with a series of conditionals.
Each guard clause can be made using a single if statement that either passes or fails; if a statement fails, execution is interrupted and the rest of the code in the block is not executed. When this is done inside a function, you will typically either return or throw an error to interrupt evaluation of subsequent conditionals.
Here is an example of how execution is prevented if any of the logic gates fail:
// Validate user-input
validate_posted();
// If the input was valid
echo 'Your data posted. Thank you for contacting us.';
function validate_posted() {
if (empty($_POST['user_name'])) {
throw new Exception('The name field can not be empty.');
}
if (empty($_POST['email'])) {
throw new Exception('The email field can not be empty.');
}
if (empty($_POST['phone'])) {
throw new Exception('The phone field can not be empty.');
}
// If everything was filled out correctly, return to the calling location
return true;
}
By using this technique, you will also be able to avoid the majority of nested if statements; in general, nesting should be avoided to make your code easier to read and understand.
Arrays instead of if, else
In a great deal of cases, you can actually just "select" the data you need to use, rather than first use conditional statements. This can both make your code more easy on the eyes to look at, and increase the performance of your application.
If you can reliably predict the content of a variable, then you might use it directly in an array to select the element of interest:
$user_roles_arr = array(
'admin' => array('edit', 'create', 'delete', 'deactivate', 'comment', 'view'),
'user' => array('edit_own', 'create', 'delete_own', 'deactivate_own', 'comment', 'view'),
'guest' => array('view')
);
Now, to select the relevant user roles we simply do like this:
$user_role = 'admin';
print_r($user_roles_arr["$user_role"]);
Instead of hard-coding the user roles, we can easily modify the above to load the roles from a database. However, for many smaller applications, hard-coding user roles is fine, as it avoids overengineering the solution.
Tell us what you think: