PHP: Shortened If, Else Statements
How to use short-hand if statements to perform conditional execution of code.
2307 views
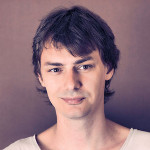
By. Jacob
Edited: 2019-12-15 18:48
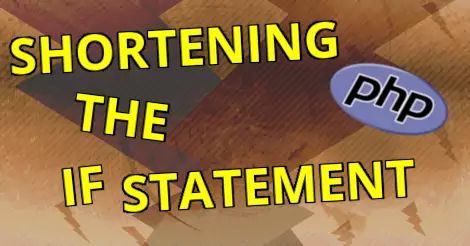
There is a few ways to write shortened if statement in PHP.
Some short-hand ways of writing if else blocks are more readable than others, and some developers therefor prefer to stick with the more readable ones.
A basic statement either returning true or false can be written like this:
$consent_given = ($_COOKIE['consent'] == 'given') ? true : false;
If statements without Curly brackets:
if ($his_name == $my_name)
echo "Hay! We got the same name!";
This example only works for a single expression, and has the disadvantage that the meaning is a bit more ambiguous. Personally, I avoid using this.
Ternary conditional operator:
$logged_in = true;
$guest = 'Viewing as Guest';
$user = 'Welcome back';
$welcome_message = $logged_in ? $user : $guest;
echo $welcome_message;
This uses the ternary operator to check if a user is logged in, and show an appropriate welcome message to the user.
Short conditional function call
$is_logged_in = false;
if (!$is_logged_in) user_role();
function user_role() {
echo '<h1>You need to be logged in to view this page</h1>';
exit();
}
Tell us what you think: