PHP: Else If Statements
How to use Else If statements in PHP, and advice on better approaches to conditional code execution.
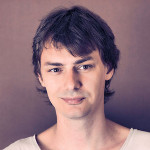
By. Jacob
Edited: 2019-12-11 10:02
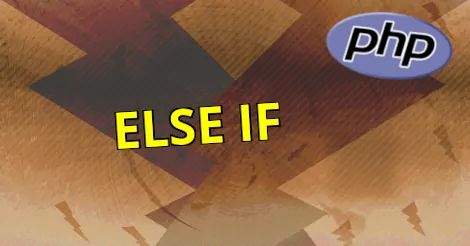
Else if statements can be used as a part of an if construct to perform conditional execution of code.
When used in PHP, you can either write each block beginning with elseif or else if. There is no difference, and whichever you choose is mostly down to personal preference.
Below is an example that uses an else if block to check for other circumstances:
if ($requested_page == '/') {
// We show the Frontpage
} else if ($requested_page == '/about') {
// We show the About Page
}
Multiple Else if blocks
You can continue to add more else if's to the same if structure, but if you have a lot of code, it may be better to dynamically call a function instead.
With if statements, it best to start with the most likely or relevant block, followed by blocks that are hit less often. This is because it takes time to check each statement before reaching the valid block is reached.
If your front page is visited more often than other pages, than it would make sense to place this as the first block to be evaluated.
if ($requested_page == 'frontpage') {
// We show the Frontpage
} else if ($requested_page == 'about') {
// We show the About Page
} else if ($requested_page == 'privacy') {
// We show the About Page
} else {
// Everything not recognized in the above Else If blocks
// Note that this last Else block is actually not needed
}
The last Else block works as a "catch-all", but it is actually not needed. We could also just place the "catch-all" block outside of the if structure.
Once a given if block evaluates to true, the rest of the blocks will not be checked, but the code after the if structure will be checked (unless you exit() from within a block).
Calling functions dynamically
When conditionally executing code, it often makes more sense to call a function rather than including a series of else if blocks. If needed, functions may also be called dynamically, and it can be more efficient than using if statements.
To call a function dynamically, we may use something like this:
$function_name = 'helloWorld';
function helloWorld(){
echo "Hallo World!";
}
$function_name();
We can do the same in OOP with a method in a class:
$method_name = "HalloWorld";
call_user_func(array($this, $method_name);
Tell us what you think: