PHP: file_get_contents vs cURL
Should you use file_get_contnts or cURL when performing HTTP requests from PHP? It does not matter; but regardless of which you use, you should still handle errors properly!
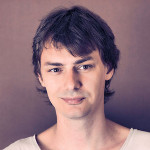
By. Jacob
Edited: 2020-08-25 09:53
I have noticed some confusion as to whether we should use cURL or file_get_contents when performing HTTP requests from PHP scripts. The truth is that it really does not matter what we use. Both are equally capable in my experience. They also seem to perform equally; but this is also one of those cases where I would not be looking at the performance to decide, since server response times is going to effect performance much more than how a request was performed.
There are actually quite a few ways to send HTTP requests from PHP, and file_get_contents and cURL is only a couple of them. It is also possible to use fopen in combination with stream_get_contents. I guess we can think of file_get_contents as a sort of catch-all, short-hand, for both reading local files and performing HTTP requests.
However, having so many different ways to do the same thing is confusing to people who are not familiar with PHP's, at times, strange syntax. For example, the fact that file- functions can also perform HTTP requests is weird and unintuitive — a new user would probably only expect them to deal with local files!
File_get_contents vs cURL vs Guzzle
I have worked with many of these methods, and also documented their use here on Beamtic, and I have not really developed a preference for one particular approach. I know there are also libraries that, supposedly, make things easier, such as guzzle for PHP — if I had to choose, then I would probably use one of these libraries, since it might also work if cURL or file- functions are not available, and they provide extra features that are not otherwise part of vanilla PHP.
Mostly I just try to stick with build-in PHP features. When it comes to simple HTTP requests, I have not really had a need to introduce outside dependencies to my code. But, if you use a framework, then you probably want to stick with whatever build-in mechanism is offered by the framework.
One benefit to using Guzzle is that it allows us to perform asynchronous and concurrent requests, something that is tediously difficult with plain PHP (although that may change in the future).
cURL and File- functions are not safe to use
As with many other PHP functions, great care should be taken to handle errors. This is something that many PHP developers are not very good at; and those of us who do handle errors still overlook things occasionally. It is not safe to simply do:
$content = file_get_contents('https://beamtic.com/api/user-agent');
Because what if the request is not completed? Well, we can check if file_get_contents failed:
if (false === ($content = file_get_contents('https://beamtic.com/api/user-agent'))) {
throw new Exception("Unable to Download web page.");
}
This is an improvement, but still not sufficient to guarantee data integrity. Now we need to also validate the server response code, and if possible, that the response body is formatted correctly before we use it. This is not something a library will help us do, so we should do our homework!
Relying on API servers outside of our own control is a potential bug waiting to happen, because even if the request itself is successful, we still can not trust that a response is well formatted.
Another example would be when just using the file- functions to access the local file system, which is also not safe. I have discussed some of the problems related to file system access here: How Safe are PHP's File Functions?; when performing a HTTP request you should also be super careful and verify that the request was successful, and that a potential response body is correctly formatted.
We should probably always validate API responses where critical.
Tell us what you think: