Determining File System Paths in Wordpress
How to correctly determine file system paths when developing for Wordpress and avoiding inconsistencies.
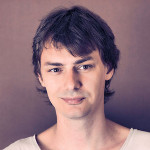
By. Jacob
Edited: 2020-12-16 21:15
Wordpress exposes just about any path needed by developers through its own internal constants and functions, so we really should not resort to using PHP to determine paths..
Wordpress already defines a constant, the ABSPATH variable, that contains the absolute path to the Wordpress installation directory. The problem with ABSPATH is that it might not be consistent enough for rare circomstances, but since we are working with Wordpress, I recommend that you stick with this regardless of potential problems.
The content of the ABSPATH constant variable might look like this:
/var/www/my-site-name/wp-content/plugins/some-plugin
In addition we also got the echo get_stylesheet_directory(); function. this will return the absolute path to the activated theme's directory. An example would be:
/var/www/my-site-name/wp-content/themes/Name-of-theme
The name of this function is rather misleading, as it has nothing to do with stylesheets. But never mind that..
Similarly, when developing Wordpress plugins, we can use echo plugin_dir_path(); to obtain the path for the plugin's directory:
/var/www/test-stay-local/wp-content/plugins/name-of-plugin/
Note. that plugin_dir_path(); produces a slash at the end. This is an inconsistency in comparison with other file system functions in Wordpress. This is just something you should be aware of.
Read: Absolute and relative paths
How is ABSPATH defined in Wordpress
Wordpress defines the ABDPATH by using dirname(__FILE__); The __FILE__ constant is a variable defined by PHP that contains the file path of the running script, and dirname returns the directory part of the path.
In Wordpress, done like this in define.php:
define('ABSPATH', dirname(__FILE__));
My first objection against using dirname() is that it might return a path that contains either backslashes or forward slashes as directory separator depending on the operating system. I would recommend that people stick to forward slashes exclusively, since this inconsistency might lead to bugs under rare circumstances. Developers also expect forward slashes; and that brings me to my next objection.
The dirname() function will return a path without a trailing slash. This means that we will not easily be able to tell if a path is a directory or a file just by looking at it. It is also confusing to developers, since they will now be asking themselves if there is a slash or not, and they might make assumptions that can lead to bugs. A returned path will look like this:
/var/www/my-site-name/wp-content/plugins/some-plugin
What I would prefer, is that the tailing slash was always included when dealing with directories. For this reason, I would rather have used something like this:
define('ABSPATH', rtrim(preg_replace('#[/\\\\]{1,}#', '/', realpath(dirname(__FILE__))), '/') . '/');
This ensures that the slash is always included, and even accounts for inconsistencies in systems in regards to adding a slash at the end (some might not add it, so we instead trim the path, and add it manually). The realpath() is also needed, since we want to account for inconsistencies between PHP-versions; for example, older versions of PHP might return a relative path instead of an absolute path, and realpath should correct that. Finally, the preg_replace will replace possible backslashes with forward slashes for increased consistency.
In a more recent version of PHP, like let us say 5.3+, this code could be simplified further:
define('ABSPATH', rtrim(preg_replace('#[/\\\\]{1,}#', '/', __DIR__), '/') . '/');
Read: Determining the Base Path in PHP
Of course we are not just Wordpress developers — we are first and foremost PHP developers — but as PHP developers we also know that determining a directory path safely can be difficult. It is not as simple as using $_SERVER['DOCUMENT_ROOT'] since this variable might not be filled out correctly on all servers.
Be mindful of inconsistencies
Once you know that Wordpress does not include a slash at directory paths, it will probably not be difficult to remember to include it manually where needed; but this just demonstrates that even something simple as working with file system paths can be confusing.
I personally prefer consistency, and since Windows also support forward slashes as a directory separator, I think we might as well just adopt it everywhere.
It should also be noted that PHP has a defined constant for the system directory separator, but I am not sure it is of much relevance since forward slash is also supported on Windows. In fact, we can even mix them without problems:
c:\some-directory/path/with\mixed\separators\
Of course we should not do that, as that can leads to bugs under some circumstances. For example, there might be a function in a random piece of third-party code that only allows using forward slashes. Backslashes will also need to be escaped before using functions like preg_replace();
Read: Determining the Base Path in PHP
In conclusion. Be mindful and try to avoid inconsistencies.
Links
- Magic constants - php.net
Tell us what you think: