PHP: cURL GET Requsts
How to perform HTTP GET requests and have the content returned using cURL in PHP.
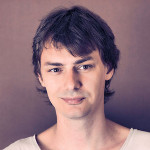
By. Jacob
Edited: 2020-01-19 14:15
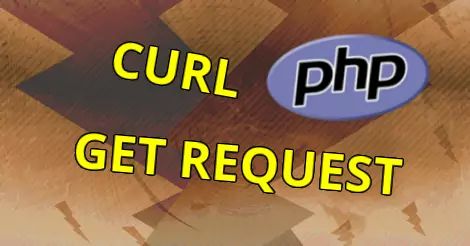
GET Requests can be performed using the cURL library using a combination of curl_init and other functions. When cURL is used from PHP, we need to use a combination of functions to specify different characteristics of the request.
Options for the request can be defined with curl_setopt before sending a request, and after loading cURL with curl_init.
To perform a HTTP GET request with cURL, and download the response body, we may do as follows:
// URL to fetch
$url = "https://beamtic.com/";
// Initialize cURL session
$ch = curl_init();
// Provide the URL for the request we are about to perform
curl_setopt($ch, CURLOPT_URL, $url);
// Option to Return the Result, rather than just true/false
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Perform the request, and save content to $result
$result = curl_exec($ch);
// Close the cURL resource, and free up system resources!
curl_close($ch);
// Shows the result!
echo $result;
Setting options for the request
Usually when someone wants to perform a GET request, they do not just want to send a HTTP request, they will also want to return the response body.
It is also possible to return the response headers, but most of the time you would just want to return the body. If you just need to return the headers, you should see this tutorial: Performing a HEAD Request with cURL
cURL has various options available via the curl_setopt function, we need to use this to tell cURL that we want to download the response body.
Normally, the curl_exec function will just return true on success or false on failure, In order to change this behavior, and instead have it return the downloaded content, we may set the CURLOPT_RETURNTRANSFER function:
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
Note. Doing this will instead make the function return the result on success and false on failure.
We may also return the HTTP response headers if we need them, to do so, we will need to set the CURLOPT_HEADER option:
curl_setopt($ch, CURLOPT_HEADER, true);
Executing the GET request
It is important that we load cURL before we set the options, so remember to first use curl_init, and finally curl_exec to perform the request.
We can perform more requests (in a loop) using the same cURL session. This might yield some performance gains when the HOST is the same, and we are just changing the relative URLs. If running in a loop, we may change the URL for subsequent requests by setting the CURLOPT_URL option again:
curl_setopt($ch, CURLOPT_URL, $new_url);
After which we can again call curl_exec to perform another request using the same session.
Finally, falling curl_close when done will free up system resources and close the session.
Tell us what you think: