Check if String Begins With String in PHP
How to check if a string begins with another string.
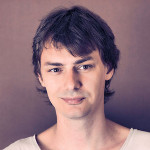
By. Jacob
Edited: 2021-02-10 15:43
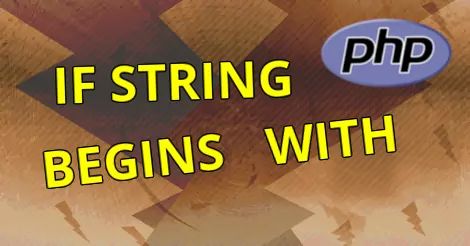
When checking if a string begins with another string, even if just a single character, we can either use a regular expression with preg_match or we can use the string functions. The string functions are usually said to be faster than using regular expressions.
It does not really matter if you use preg_match or stripos, since the speed difference between then is truly minuscule.
The following code checks if a given string contains double dash "//" at the beginning, or rather, at position zero "0". The stripos function will return the position of the string on success, and false if the string was not found:
$str = '//something/more';
if (stripos($str, '//') === 0) {
echo 'The string contains double dash at the beginning.';
} else {
echo 'The string did not begin with double dash.';
}
exit();
From PHP 8 and onwards, we can use the str_starts_with function:
if(str_starts_with('PHP is cool', 'PHP')) {
echo 'The string begins with PHP';
}
Using a regular expression
Regular expressions may be a tiny bit slower, although this will probably not matter in the majority of cases. I would use whatever I was most comfortable using.
We may also use preg_match to check if a string begins with another string, to do so, we should use a pattern like this /^something/, the "^" caret character marks the beginning of the string.
But, since we are looking for double dashes, we should really use this /^\/\//. Note the backslash characters "\" in front of the forward slashes "/"; these are needed to escape the forward slash, since it has a special meaning in regular expressions.
if (preg_match('/^\/\//', $str)) {
return 'The string contains double dash at the beginning.';
} else {
return 'The string did not begin with double dash.';
}
Note. PHP has a function called preg_quote for escaping characters in regular expressions; using this is easier than manually escaping special characters.
The preg_quote function should only be used on the relevant parts of the regular expression:
$string = preg_quote('//');
if (preg_match('/^'. $string .'/', $str)) {
return 'The string contains double dash at the beginning.';
} else {
return 'The string did not begin with double dash.';
}
Tell us what you think: