Complex (curly) Syntax in PHP
A tutorial on using complex curly syntax to access arrays and objects in strings.
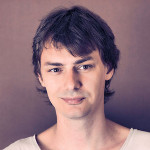
By. Jacob
Edited: 2021-02-13 21:39
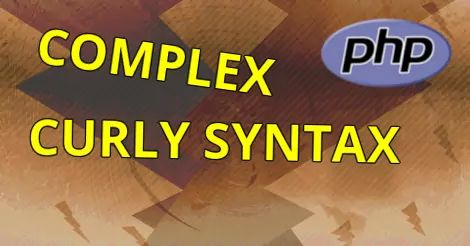
Complex (curly) syntax is a term used to describe the use of ${ } (dollar sign curly bracket) around variables and objects in PHP. The syntax may be used to explicitly tell PHP when a variable name starts and ends, which is useful when accessing arrays and objects in strings, as well as when working with variable variables.
When using complex curly syntax we may access arrays by key inside of heredocs, something that is not possible without curly braces.
We may also create variable names dynamically, also known as variable variables, and we can even use special characters as variable names. To assign a variable dynamically, we may do like this:
$variable_name = 'name_of_variable'; // Name the variable
${$variable_name} = 'some data'; // Assign some data
echo ${'name_of_variable'}; // Output the content
The below example uses an array in a heredoc string, very useful for making HTML templates:
$content = <<<MY_HEREDOC
{$hallo['here_goes']}
MY_HEREDOC;
The same syntax can also be used when accessing methods in heredocs:
$content = <<<MY_HEREDOC
{$myObject->hallo_world()}
MY_HEREDOC;
And when accessing complex property names of objects:
$test_object = (object) [];
$test_object->{'property name with spaces'} = 'some value';
Accessing functions with complex curly syntax
We can not currently access the return value of a normal function. This does seem like a flaw or inconsistency in the language, but the question is if we really need this anyway.
Using heredocs for templates is fine until we start to include complex PHP, such as calling function and methods within the heredoc.
The whole idea in maintaining template files is to keep your HTML apart from your PHP code. For this, heredocs is somewhat a compromise. Many editors will not syntax-highlight the HTML in the heredoc, which might introduce coding-errors that are hard to spot. This is, however, not a big problem.
A big problem is when a template requires complex conditional logic, or when we start to include calls to functions and methods. If we do this, then there is a risk that the code is becoming harder to maintain.
Instead we may choose to load application-specific .php files that handles generation of template content. Including (but not limited to):
- load data from database
- iterate over data
- create site menus.
- generate HTML lists or tables.
Doing this is extremely powerful vs mixing your HTML with PHP-code. You could even keep the HTML in separate .html files and then do a simple RegEx replacement before sending a HTTP response back to the client.
Note. If you are working with HTML templates and want the ability to access functions, you can create a wrapper object for the functions. Alternatively, you can also use a template language. But, both of these solutions may be counterproductive in the long-term.
Links
- Complex (curly) syntax - php.net
Tell us what you think: