A Grep Equivalent in PHP
This shows how to do a grep-like action using regular expressions from a PHP script.
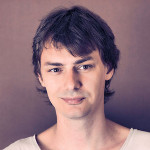
By. Jacob
Edited: 2020-03-08 13:04
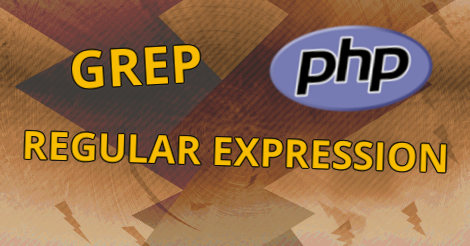
If you have been using Linux long enough you will probably also have used the grep command. Grep allows us to easily filter out irrelevant entries in log files, and only return the things we are interested in. There is no grep in PHP, but we can easily create a similar function.
Note. There is also a modified version of grep called zgrep, this can be used to read .gz compressed log files.
There are two simple ways have grep like functionality from within PHP, one of them is by using shell_exec() and calling grep directly, the other is by using a regular expression.
I prefer using a RegEx personally, since it avoids shell commands, and is actually not very hard. I.e:
$system_log = file_get_contents("/var/log/syslog");
preg_match_all("|([^\n]+.*CRON.*)|", $system_log, $out, PREG_PATTERN_ORDER); // A RegEx grep in PHP
$cron_log_array = $out[0]; // $out[0] contains the content matched by the RegEx
foreach($cron_log_array as &$log_entry) {
echo "\n$log_entry"; // "\n" is newline
}
Permissions on system files
Beware that certain files, such as syslog, needs root access for you to read them. This means you can not call a PHP script over HTTP and have it read the file. At least not without changing the permissions of the file, or giving your web-server root access (extremely bad idea).
I do not recommend you give your web server access to read those files, as it would be bad for security reasons. But, you can still read the files by calling PHP scripts locally on the server as root. This is much more secure, since no one from the outside would be able to directly mess with system files, if they manage to find a security hole in your web based app or website.
You can even setup a cron job that runs the script via PHP locally, avoiding the use of cURL to run the script. I often see people use cURL to run local scripts, which can cause problems if you loose the internet/lan connection, and it is really not necessary!
With that out of the way, let us focus on finding a solution :-)
Using grep in PHP scripts
If you want to avoid the use of shell_exec(), you can use preg_match_all() instead:
$system_log = file_get_contents("/var/log/syslog");
preg_match_all("|([^\n]+.*CRON.*)|", $system_log, $out, PREG_PATTERN_ORDER); // A RegEx grep in PHP
$cron_log_array = $out[0]; // $out[0] contains the content matched by the RegEx
foreach($cron_log_array as &$log_entry) {
echo "\n$log_entry"; // "\n" is newline
}
This script reads the syslog file, but it can also be used for other files.
A reguler expression "greps" all the lines in the syslog file containing "CRON".
The regular expression: ([^\n]+.*CRON.*) only looks for newlines and the part you want it to match, "CRON". Simply replace "CRON" with whatever you want it to look for.
I do not recommend using shell_exec(), since it might be disabled for security reasons on some servers. But using it would give you direct access to grep, avoiding the use of RegEx:
$cron_log = shell_exec("grep CRON /var/log/syslog");
header("Content-Type: text/plain; charset=utf-8");
echo $cron_log;
When using shell_exec(), it will return the output of the grep command as a string. You can then do further work on this string. If you want to convert it into an array, simply use the explode() function.
$cron_log_array = explode("\n", $cron_log);
header("Content-Type: text/plain; charset=utf-8");
foreach($cron_log_array as &$cron_job) {
echo "\n$cron_job";
}
Tell us what you think: