PHP: The Else Clause is Rarely Needed
The else clause in if statements is usually not required, but developers include it anyway because, that is just the way they code.
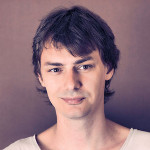
By. Jacob
Edited: 2020-05-30 01:18
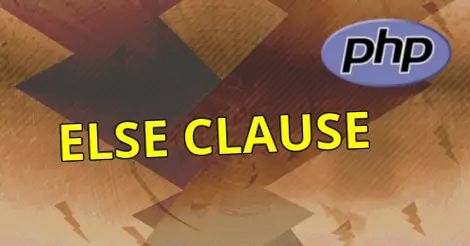
The use of a final else clause in if statements is rarely really needed, but we use it anyway because of the way we think. Interestingly, I always seem to be able to refactor code in a way that does not require a final else clause—and this is often both cleaner and easier to read.
The final else clause is only needed when you want the code inside of the else block to know whether the preceding if condition'(s) turned out to be true. I personally prefer to avoid the final else to simplify and clean up my code—I even avoid using else if's unless there is logical reason to use them.
In order for the else clause to be required, the following needs to be true:
- The code inside the else clause needs to be able to deduce the state of preceding conditions.
- The else block must not perform exit or return.
- The code after the if statement must finish executing.
Even then, you can probably still find a way to reverse your thinking, and avoid the else block entirely. I will try to propose a situation where this is not possible.
The below example requires the else block to call the event logger, as well as a function that returns the username:
// More code above this point
if ($user_handler->login_check()) {
$message = 'Welcome ' . $user_handler->user_name();
} else {
$message = 'A login check has failed. What are you doing? This event has been logged.';
$logger->event('Warning: Possible session hijack attempt! A user failed a loin check. This should not happen.', $client_info_object);
}
// Continue executing...
echo $message;
Reversing our thinking does not seem to work for avoiding the else clause in this case, for these reasons:
- The user_name(); method must be called after performing the login_check.
- The rest of the script still needs to finish executing.
If we reversed our logic, then it would mean checking the login for failure rather than for success, and moving the user_name(); outside of the comfort of the if blocks.
Of course, you could just define the $message variable before performing the login check, but that might throw an exception, depending on how user_name(); is implemented.
Avoiding the Else clause
I am in favor of leaving out the else clause in most cases, especially when it is not needed. You probably do not want to spend time refactoring existing code that works, but if you have an else block somewhere, then you can probably refactor your code in a way that does not use it.
I think the reason why its use is so widespread is because a lot of programming examples include it, and it might be the way people have learned to think when coding, similar to how they write:
if ($some_var == false) {
// ...
}
Instead of:
if (false == $some_var) {
// ...
}
Which lowers the chance of typos and accidentally assigning a value instead of performing a comparison.
I am not a 100% ready to declare else blocks redundant, as there may be the rare cases where it is the simplest solution. Besides, some of these things are more down to personal preference. Some developers might use it because they feel that it makes it easier for them to understand the code.
Else if and conditional code execution
We can often easily refactor else if's into multiple if's. That does not mean that we should always do it, since using else if is theoretically more efficient when you need to switch between code conditionally, and when subsequent checks does not need to be performed.
But, in that case, we could also use a switch, which in theory should be more efficient than an if statement. This is premature optimization, of course, but even still, I think it is fine to develop good coding habits.
If the code that needs to run is very large, then I prefer dynamically loading the code that needs to run. This will both save system resources as well as make your code easier to maintain. There is at least a few ways you can do this:
- You can dynamically instantiate a class by a variable and rely on its __construct(); method to run OO PHP code.
- You can call a function or method dynamically using a variable.
- You can dynamically include a file by a variable.
This is also about personal preference—but there is clearly some techniques that are going to be more efficient than others.
Tell us what you think: