PHP: Using the Ternary Operator
Using the ternary operator in PHP to perform shorthand comparisons and save space in our code.
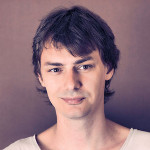
By. Jacob
Edited: 2020-07-25 01:00
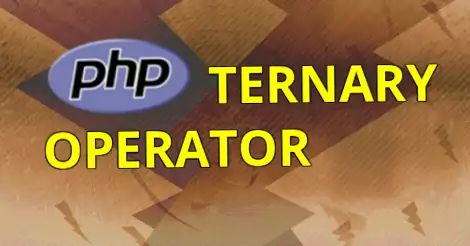
The ternary operator ? is useful for performing conditional execution of code, and for performing basic shorthand comparisons in PHP; indeed, in some cases a five line if statement can be turned into a nice one-liner that saves space in your code. The downside is that it may come at a small cost of code readability.
The cool thing about the ternary operator is that it allows us to both assign a value to a variable, and perform an if else in a single line of code.
For example, define a variable based on whether a suberglobal was used:
$name = (isset($_POST['name'])) ? $_POST['name'] : false;
The above is basically a shorthand version of the if statement. When working with superglobals that contain user input, it is considered good practice to check if variables are set before using them, therefor we might have a lot of isset() in our code. Traditionally that might have looked like this:
if (false === isset($_POST['name'])) {
return false;
}
echo $_POST['name'];exit();
This is a minimal example using a simplified if structure that avoids the else clause entirely; but sometimes you might save five or more lines of code — how much you save depends on the complexity of your if statement.
Simplifying if statements is a great way to increase the readability of your code; and better readability will often lead to faster understanding.
Is the ternary operator hard to read?
Whether the ternary operator is hard to read will depend mostly on familiarity; if a developer knows about it, then they will probably find it easier to read than if/else blocks, simply because there is less mental decoding required.
The most difficult part is remembering the position of the parts used to construct the statement.
$name = (isset($_POST['name'])) ? $_POST['name'] : false;
This would define the $name variable as false when the POST variable is not set, or as the POST variable when it is set.
An easy way to remember where the part goes, is by thinking of the traditional if/else statement; first comes the if then comes what happens if the statement was true, and finally comes the else block.
Null coalescing operator
There is an even easier way to perform these kinds of checks, by using the null coalescing operator:
$_POST['name'] ?? false; // Sets name to false if it is not defined
Tell us what you think: