Removing Elements From PHP Arrays
How to easily remove elements from arrays in PHP using the unset function.

Edited: 2021-03-07 10:03
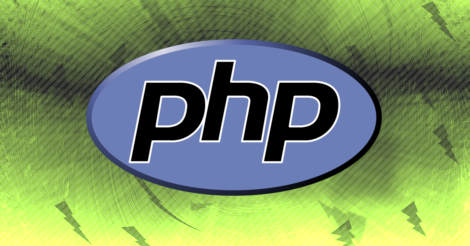
Removing elements from arrays can be done with the unset() function. If you do not know the array key of the element you wish to remove, and you only know the value, you could use a combination of functions to find the key.
If you do know the key:
$my_array = array(
'boss' => 'Jacob',
'janitor' => 'Kim'
'worker' => 'Jones'
);
$key = 'janitor';
unset($my_array["$key"]);
Remember, if your key is a number, quotes are not needed:
$key = 22; // String or Int
If you do not know the key, but you know the value, you could use array_flip(), and then unset via the flipped value:
$my_array = array(
'boss' => 'Jacob',
'janitor' => 'Kim'
'worker' => 'Jones'
);
$my_array = array_flip($my_array);
unset($my_array["$key"]);
$my_array = array_flip($my_array); // Reversing the flip
print_r($my_array);
Another option is to use array_search():
$my_array = array(
'boss' => 'Jacob',
'janitor' => 'Kim'
'worker' => 'Jones'
);
$key = array_search('Jacob', $my_array); // $key = boss
unset($my_array["$key"]);
It is generally considered good practice to always use quotes when working with keys. If you are using an array element, use curly brackets:
$key = 'The Key'; // String or Int
unset($my_array["{$array['MyKey']}"]);
Removing duplicate values
Duplicated values can be removed using array_keys with array_flip, which is more simple than iterating over an array, and faster than using the dedicated array_unique function.
We could just double flip the array, but this would result in "missing keys", which is less than ideal when other developers might be iterating over the array without our knowledge. Using array_keys avoids this problem by re-generating the keys.
$some_array = array('HTML', 'CSS', 'JavaScript', 'PHP', 'HTML', 'HTML', 'Jquery');
$unique_array = array_keys(array_flip($some_array));
print_r($unique_array);
Output:
Array
(
[0] => HTML
[1] => CSS
[2] => JavaScript
[3] => PHP
[4] => Jquery
)
Tell us what you think: